二叉树的括号表示法作为函数参数,构造一颗二叉树,使用二叉链存储结构,包括输入、查找节点、先序中序后序遍历,并求出二叉树的高,并且销毁二叉树的代码
时间: 2024-06-19 11:03:28 浏览: 13
二叉树的括号表示法是一种用字符串来表示二叉树的方法,具体来说,对于一个节点,它的表示法为:(左子树)(右子树)节点值。如果它的左子树为空,则用"()"代替,如果右子树为空,则只保留左子树的表示法。下面是使用括号表示法构造一颗二叉树的示例代码:
```c++
#include<iostream>
#include<stack>
using namespace std;
struct TreeNode{
char val;
TreeNode* left;
TreeNode* right;
TreeNode(char x): val(x), left(nullptr), right(nullptr){};
};
void build_tree(TreeNode*& root, string s){
stack<TreeNode*> st;
for(int i=0;i<s.size();i++){
if(s[i] == '(') continue;
else if(s[i] == ')') st.pop();
else{
TreeNode* node = new TreeNode(s[i]);
if(root == nullptr) root = node;
else{
TreeNode* parent = st.top();
if(parent->left == nullptr) parent->left = node;
else parent->right = node;
}
st.push(node);
}
}
}
void pre_order(TreeNode* root){
if(root == nullptr) return;
cout << root->val << " ";
pre_order(root->left);
pre_order(root->right);
}
void in_order(TreeNode* root){
if(root == nullptr) return;
in_order(root->left);
cout << root->val << " ";
in_order(root->right);
}
void post_order(TreeNode* root){
if(root == nullptr) return;
post_order(root->left);
post_order(root->right);
cout << root->val << " ";
}
TreeNode* find_node(TreeNode* root, char x){
if(root == nullptr) return nullptr;
if(root->val == x) return root;
TreeNode* left = find_node(root->left, x);
if(left != nullptr) return left;
return find_node(root->right, x);
}
int get_height(TreeNode* root){
if(root == nullptr) return 0;
int left_height = get_height(root->left);
int right_height = get_height(root->right);
return max(left_height, right_height) + 1;
}
void destroy_tree(TreeNode*& root){
if(root == nullptr) return;
destroy_tree(root->left);
destroy_tree(root->right);
delete root;
root = nullptr;
}
int main(){
string s = "(((A)B(C))D((E(F))G))";
TreeNode* root = nullptr;
build_tree(root, s);
cout << "Preorder traversal: ";
pre_order(root);
cout << endl;
cout << "Inorder traversal: ";
in_order(root);
cout << endl;
cout << "Postorder traversal: ";
post_order(root);
cout << endl;
char x = 'E';
TreeNode* node = find_node(root, x);
if(node == nullptr) cout << "Node " << x << " not found!" << endl;
else cout << "Node " << x << " found!" << endl;
int height = get_height(root);
cout << "Height of tree: " << height << endl;
destroy_tree(root);
return 0;
}
```
相关推荐
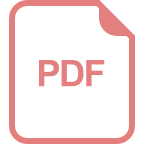
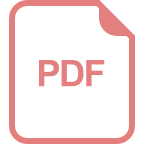
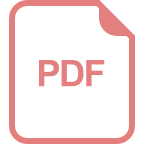














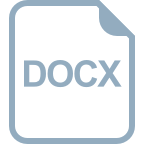
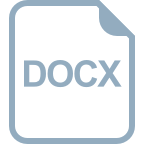