请用标准c语言构建一个二叉树,要求使用括号表示法输入二叉树并转化为二叉树的链式存储结构;横向输出二叉树;求二叉树的高度;统计二叉树中的节点个数;中序遍历该二叉树(采用递归算法);层序遍历该二叉树
时间: 2023-11-14 13:30:01 浏览: 41
好的,我来逐一回答你的问题。
首先是构建二叉树。以下是用标准 C 语言实现的二叉树结构体:
```c
typedef struct node {
char data; // 节点数据
struct node *lchild; // 左子节点
struct node *rchild; // 右子节点
} Node, *Tree;
```
接下来是用括号表示法输入二叉树并转化为二叉树的链式存储结构。输入格式如下:
```
(A(B(D,E),C(F,G)))
```
这个表示一个如下图所示的二叉树:
```
A
/ \
B C
/ \ / \
D E F G
```
下面是转化为链式存储结构的代码:
```c
#include <stdio.h>
#include <stdlib.h>
Node *create_tree(char *str, int *index) {
Node *root = NULL;
if (str[*index] != '\0' && str[*index] != ')') {
root = (Node *)malloc(sizeof(Node));
root->data = str[*index];
root->lchild = NULL;
root->rchild = NULL;
(*index)++;
if (str[*index] == '(') {
(*index)++;
root->lchild = create_tree(str, index);
}
if (str[*index] == ',') {
(*index)++;
root->rchild = create_tree(str, index);
}
}
(*index)++;
return root;
}
int main() {
char str[100];
int index = 0;
scanf("%s", str);
Tree root = create_tree(str, &index);
return 0;
}
```
这个函数的返回值是一个二叉树的根节点指针。
接下来是横向输出二叉树。我们可以通过中序遍历二叉树,同时记录每个节点在树中的位置,最后按行输出。这里使用了一个辅助函数 `tree_height` 来计算二叉树的高度:
```c
int tree_height(Tree root) {
if (root == NULL) {
return 0;
}
int left_height = tree_height(root->lchild);
int right_height = tree_height(root->rchild);
return left_height > right_height ? left_height + 1 : right_height + 1;
}
void print_tree(Tree root, int level, int pos[]) {
if (root == NULL) {
return;
}
pos[level] = pos[level - 1] * 2;
print_tree(root->lchild, level + 1, pos);
printf("%*c%c\n", pos[level] - pos[level - 1], ' ', root->data);
pos[level] = pos[level - 1] * 2 + 1;
print_tree(root->rchild, level + 1, pos);
}
void print(Tree root) {
int height = tree_height(root);
int pos[height];
for (int i = 0; i < height; i++) {
pos[i] = 1;
}
print_tree(root, 1, pos);
}
```
接下来是求二叉树的高度。这个函数非常简单,就是递归计算左子树和右子树的高度,然后取较大值加 1:
```c
int tree_height(Tree root) {
if (root == NULL) {
return 0;
}
int left_height = tree_height(root->lchild);
int right_height = tree_height(root->rchild);
return left_height > right_height ? left_height + 1 : right_height + 1;
}
```
接下来是统计二叉树中的节点个数。这个函数也非常简单,就是递归计算左子树和右子树的节点数,然后加上 1:
```c
int node_count(Tree root) {
if (root == NULL) {
return 0;
}
int left_count = node_count(root->lchild);
int right_count = node_count(root->rchild);
return left_count + right_count + 1;
}
```
接下来是中序遍历该二叉树(采用递归算法)。中序遍历的顺序是左子树 -> 根节点 -> 右子树,按照这个顺序递归即可:
```c
void inorder_traversal(Tree root) {
if (root == NULL) {
return;
}
inorder_traversal(root->lchild);
printf("%c ", root->data);
inorder_traversal(root->rchild);
}
```
最后是层序遍历该二叉树。层序遍历需要借助队列来实现。具体实现方法是:先将根节点入队,然后每次从队列中取出一个节点,输出它的值,并将它的左右子节点入队。直到队列为空为止:
```c
void level_order_traversal(Tree root) {
if (root == NULL) {
return;
}
Queue queue;
init_queue(&queue);
enqueue(&queue, root);
while (!is_empty(&queue)) {
Node *node = dequeue(&queue);
printf("%c ", node->data);
if (node->lchild != NULL) {
enqueue(&queue, node->lchild);
}
if (node->rchild != NULL) {
enqueue(&queue, node->rchild);
}
}
}
```
以上就是用标准 C 语言实现二叉树的一些常见操作的代码。
相关推荐
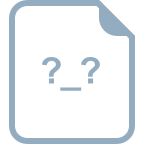
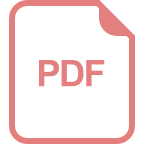














