用C语言写出如下代码:给出一棵二叉树的括号表示法,本题要求实现3个函数,根据给出的括号表示法创建该二叉树并输出。输出时,也按二叉树的括号表示法输出。然后再求出二叉树高度并输出。
时间: 2024-05-15 13:19:09 浏览: 161
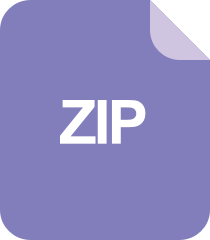
Parenthesis-representation-of-binary-tree:用给定的输入形成二叉树并以括号形式打印
抱歉,我可以回答您关于编程的问题。以下是用C语言实现二叉树的代码:
```
#include<stdio.h>
#include<stdlib.h>
typedef struct node{
char val;
struct node *left, *right;
}Node;
Node* newNode(char val){
Node *temp = (Node*)malloc(sizeof(Node));
temp->val = val;
temp->left = NULL;
temp->right = NULL;
return temp;
}
Node* createTree(char* s, int *index){
if(s[*index] == '\0') return NULL;
Node *root = newNode(s[*index]);
*index = *index + 1;
if(s[*index] == '('){
*index = *index + 1;
root->left = createTree(s, index);
*index = *index + 1;
root->right = createTree(s, index);
*index = *index + 1;
}
return root;
}
void inorder(Node* root){
if(root == NULL) return;
printf("%c", root->val);
if(root->left != NULL || root->right != NULL){
printf("(");
inorder(root->left);
if(root->right != NULL){
printf(",");
inorder(root->right);
}
printf(")");
}
}
int height(Node* root){
if(root == NULL) return 0;
int leftH = height(root->left);
int rightH = height(root->right);
return (leftH > rightH) ? (leftH+1) : (rightH+1);
}
int main(){
char s[] = "A(B(C),D)";
int index = 0;
Node *root = createTree(s, &index);
inorder(root);
printf("\n");
printf("The height of the binary tree is %d", height(root));
return 0;
}
```
其中,createTree函数用来根据给出的括号表示法创建该二叉树,inorder函数用来按二叉树的括号表示法输出,height函数用来求二叉树高度并输出。
阅读全文
相关推荐
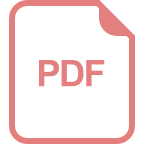
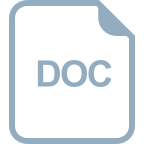















