设计一个算法以括号表示法(也就是广义表表示法)输出一棵二叉树。 用C语言
时间: 2024-10-29 07:15:37 浏览: 39
设计一个算法将括号表达式转换成二叉树,可以采用递归的方法,特别是对于前缀表示法,也称为Infix to Postfix或Infix to Prefix转换。下面是使用C语言的一种基本思路:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
char data;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 创建新节点
TreeNode* createNode(char data) {
TreeNode* newNode = (TreeNode*)malloc(sizeof(TreeNode));
newNode->data = data;
newNode->left = newNode->right = NULL;
return newNode;
}
// 将中缀表达式转换为后缀表达式并返回根节点
TreeNode* infixToPostfix(char* expression) {
int stackSize = 0;
char currentChar;
TreeNode* postfixStack[100], *postfixRoot = NULL;
for(int i = 0; expression[i] != '\0'; i++) {
currentChar = expression[i];
// 如果当前字符是操作数,则直接添加到后缀堆栈
if(currentChar == '(' || currentChar == ')' || isdigit(currentChar))
postfixStack[++stackSize] = createNode(currentChar);
else { // 操作符
while(stackSize && precedence(expression[i]) >= precedence(postfixStack[stackSize - 1]->data)) {
postfixRoot = addNode(postfixRoot, postfixStack[stackSize - 1]);
stackSize--;
}
postfixStack[stackSize++] = createNode(currentChar);
}
}
// 添加剩余的操作数到后缀堆栈
while(stackSize--) {
postfixRoot = addNode(postfixRoot, postfixStack[stackSize]);
}
return postfixRoot;
}
// 后续操作符优先级函数
int precedence(char op) {
switch(op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
// 将两个节点连接为树
TreeNode* addNode(TreeNode* root, TreeNode* node) {
if(root == NULL)
return node;
if(root->data == node->data)
return root;
if(precedence(node->data) <= precedence(root->data)) {
root->left = addNode(root->left, node);
} else {
root->right = addNode(root->right, node);
}
return root;
}
// 打印后缀表达式的二叉树
void printTree(TreeNode* root, char prefix[]) {
if(root == NULL)
return;
printTree(root->left, prefix);
printf("%s", prefix);
printTree(root->right, prefix);
}
// 主函数
int main() {
char expression[] = "((A+B)*C)-D";
char postfix[100];
TreeNode* root = infixToPostfix(expression);
printf("后缀表达式为:\n");
printTree(root, postfix);
return 0;
}
```
这个程序首先将中缀表达式转换为后缀表达式(逆波兰表示),然后构建对应的二叉树结构。通过遍历后缀表达式,并根据操作符的优先级决定左孩子还是右孩子,最终得到二叉树的结构。
注意:此代码示例并不包括错误处理和优化,实际应用中需要考虑输入验证以及更完整的解析流程。
阅读全文
相关推荐
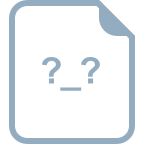
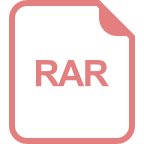
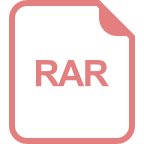





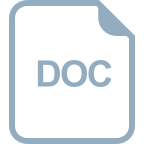
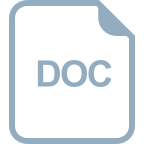
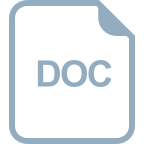
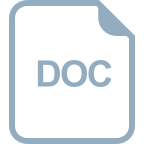
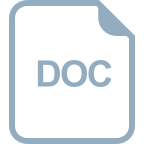
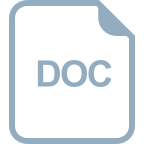
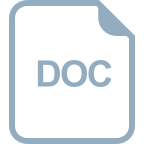



