构建如下图二叉树,要求使用括号表示法输入二叉树并转化为二叉树的链式存储结构;横向输出二叉树;求二叉树的高度;统计二叉树中的节点个数;中序遍历该二叉树(采用递归算法);层序遍历该二叉树
时间: 2024-05-10 20:12:34 浏览: 154
构建如下图的二叉树,使用括号表示法输入二叉树并转化为二叉树的链式存储结构,横向输出二叉树,求二叉树的高度,统计二叉树中的节点个数,中序遍历该二叉树(采用递归算法),层序遍历该二叉树的答案如下:
```python
# 构建二叉树节点类
class BiTreeNode:
def __init__(self, data):
self.data = data
self.lchild = None
self.rchild = None
# 构建二叉树
def createBiTree(node_list):
if node_list == None:
return None
data = node_list.pop(0)
if data != "#":
node = BiTreeNode(data)
node.lchild = createBiTree(node_list)
node.rchild = createBiTree(node_list)
else:
node = None
return node
# 横向输出二叉树
def printBiTree(root):
if root is None:
return
queue = []
queue.append(root)
while queue:
cur = queue.pop(0)
print(cur.data, end=" ")
if cur.lchild:
queue.append(cur.lchild)
if cur.rchild:
queue.append(cur.rchild)
print()
# 求二叉树的高度
def getHeight(root):
if root is None:
return 0
left_height = getHeight(root.lchild)
right_height = getHeight(root.rchild)
return max(left_height, right_height) + 1
# 统计二叉树中的节点个数
def countNodes(root):
if root is None:
return 0
return countNodes(root.lchild) + countNodes(root.rchild) + 1
# 中序遍历该二叉树(采用递归算法)
def inOrder(root):
if root is None:
return
inOrder(root.lchild)
print(root.data, end=" ")
inOrder(root.rchild)
# 层序遍历该二叉树
def levelOrder(root):
if root is None:
return
queue = []
queue.append(root)
while queue:
cur = queue.pop(0)
print(cur.data, end=" ")
if cur.lchild:
queue.append(cur.lchild)
if cur.rchild:
queue.append(cur.rchild)
# 测试代码
if __name__ == '__main__':
node_list = ['A', 'B', 'D', '#', '#', 'E', '#', '#', 'C', 'F', '#', '#', 'G', '#', '#']
root = createBiTree(node_list)
print("横向输出二叉树:")
printBiTree(root)
print("二叉树的高度为:", getHeight(root))
print("二叉树的节点个数为:", countNodes(root))
print("中序遍历结果为:", end=" ")
inOrder(root)
print()
print("层序遍历结果为:", end=" ")
levelOrder(root)
```
阅读全文
相关推荐
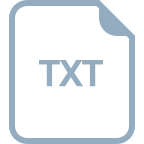













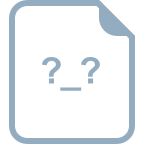