用c语言实现使用栈将一个很长(>30)的十进制数转换为二进制数
时间: 2024-04-17 12:25:17 浏览: 213
可以使用栈来实现将一个很长的十进制数转换为二进制数。下面是用C语言实现的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
struct Stack {
int top;
unsigned capacity;
int* array;
};
struct Stack* createStack(unsigned capacity) {
struct Stack* stack = (struct Stack*)malloc(sizeof(struct Stack));
stack->capacity = capacity;
stack->top = -1;
stack->array = (int*)malloc(stack->capacity * sizeof(int));
return stack;
}
int isFull(struct Stack* stack) {
return stack->top == stack->capacity - 1;
}
int isEmpty(struct Stack* stack) {
return stack->top == -1;
}
void push(struct Stack* stack, int item) {
if (isFull(stack)) {
printf("Stack is full.\n");
return;
}
stack->array[++stack->top] = item;
}
int pop(struct Stack* stack) {
if (isEmpty(stack)) {
printf("Stack is empty.\n");
return -1;
}
return stack->array[stack->top--];
}
void decimalToBinary(long long decimal) {
struct Stack* stack = createStack(MAX_SIZE);
while (decimal > 0) {
push(stack, decimal % 2);
decimal = decimal / 2;
}
printf("Binary representation: ");
while (!isEmpty(stack)) {
printf("%d", pop(stack));
}
}
int main() {
long long decimal;
printf("Enter a decimal number: ");
scanf("%lld", &decimal);
decimalToBinary(decimal);
return 0;
}
```
上述代码使用了一个栈来存储二进制数的每一位。首先,我们将十进制数从右往左依次除以2,并将余数(0或1)入栈。然后,从栈中依次弹出元素,即可得到二进制表示。
请注意,此代码中使用了一个自定义的栈结构体和相关的操作函数来实现栈的基本功能。你可以根据需要进行修改和扩展。
阅读全文
相关推荐
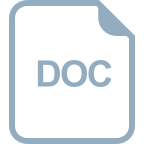
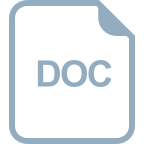
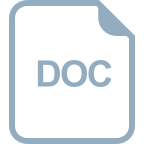




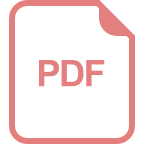


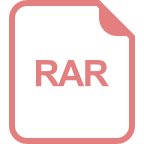
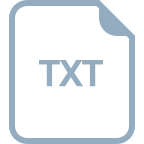
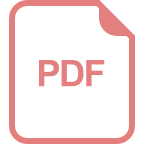
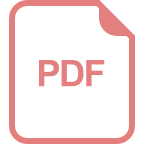

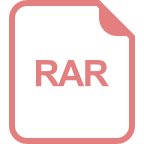
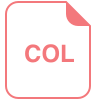
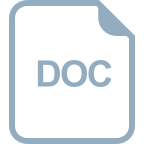