java用类和对象完成编写一个程序用来统计十个同学的最高分,最低分和平均分。
时间: 2024-11-27 20:14:02 浏览: 4
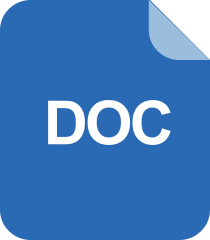
最高分、最低分、平均分

在Java中,我们可以创建一个`Student`类来表示每个学生,包含他们的分数属性。然后,我们可以创建一个`ScoreStatistics`类来处理计算最高分、最低分和平均分的功能。这里是一个简单的例子:
```java
// Student 类,代表一个学生及其分数
class Student {
private String name;
private int score;
public Student(String name, int score) {
this.name = name;
this.score = score;
}
// 获取分数的方法
public int getScore() {
return score;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", score=" + score +
'}';
}
}
// ScoreStatistics 类,用于统计最高分、最低分和平均分
class ScoreStatistics {
private List<Student> students;
public ScoreStatistics(List<Student> students) {
this.students = students;
}
public void calculateScores() {
if (students.isEmpty()) {
System.out.println("No students to calculate scores.");
return;
}
int highestScore = Collections.max(students.stream().mapToInt(Student::getScore).boxed());
int lowestScore = Collections.min(students.stream().mapToInt(Student::getScore).boxed());
double sum = students.stream().mapToDouble(Student::getScore).sum();
double averageScore = sum / students.size();
System.out.println("Highest score: " + highestScore);
System.out.println("Lowest score: " + lowestScore);
System.out.println("Average score: " + averageScore);
}
}
public class Main {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("Alice", 95));
students.add(new Student("Bob", 88));
// ... 其他八个同学的添加类似
ScoreStatistics stats = new ScoreStatistics(students);
stats.calculateScores();
}
}
```
阅读全文
相关推荐
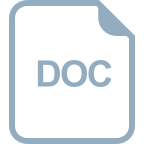
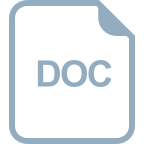














