yolov5添加BiFPN
时间: 2023-09-15 12:22:05 浏览: 366
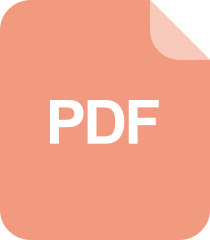
改进YOLOv5-添加Bifpn
对于YOLOv5模型,添加BiFPN(Bilateral Feature Pyramid Network)可以提高其精度和鲁棒性。BiFPN是一种用于目标检测任务的特征金字塔网络结构,通过在不同层级的特征金字塔上引入双向的信息流动,有效地融合多尺度的特征信息,提升目标检测性能。
要在YOLOv5中添加BiFPN,可以按照以下步骤进行操作:
1. 导入所需的库和模块:
```python
import torch
import torch.nn as nn
from models.common import Conv
```
2. 定义BiFPN类:
```python
class BiFPN(nn.Module):
def __init__(self, num_channels, num_layers):
super(BiFPN, self).__init__()
self.num_channels = num_channels
self.num_layers = num_layers
self.up_convs = nn.ModuleList([Conv(num_channels, num_channels, 1) for _ in range(num_layers)])
self.down_convs = nn.ModuleList([Conv(num_channels, num_channels, 1) for _ in range(num_layers)])
self.p6_up = Conv(num_channels, num_channels, 1)
self.p7_down = Conv(num_channels, num_channels, 1)
self.swish = nn.SiLU()
```
3. 实现BiFPN的前向传播方法:
```python
def forward(self, inputs):
p3, p4, p5, p6, p7 = inputs
# Bottom-up pathway
p7_td = self.p7_down(p7)
p6_td = self.p6_up(p6) + nn.functional.interpolate(p7_td, scale_factor=2, mode='nearest')
p5_td = self.up_convs[0](p5) + nn.functional.interpolate(p6_td, scale_factor=2, mode='nearest')
p4_td = self.up_convs[1](p4) + nn.functional.interpolate(p5_td, scale_factor=2, mode='nearest')
p3_out = self.up_convs[2](p3) + nn.functional.interpolate(p4_td, scale_factor=2, mode='nearest')
# Top-down pathway
p4_out = self.down_convs[0](p4) + nn.functional.interpolate(p3_out, scale_factor=0.5, mode='nearest')
p5_out = self.down_convs[1](p5) + nn.functional.interpolate(p4_out, scale_factor=0.5, mode='nearest')
p6_out = self.down_convs[2](p6) + nn.functional.interpolate(p5_out, scale_factor=0.5, mode='nearest')
p7_out = self.p7_down(p7) + nn.functional.interpolate(p6_out, scale_factor=0.5, mode='nearest')
return p3_out, p4_out, p5_out, p6_out, p7_out
```
4. 在YOLOv5的主干网络中调用BiFPN:
```python
class YOLOv5(nn.Module):
def __init__(self, num_classes):
super(YOLOv5, self).__init__()
# Define the backbone network
# Add BiFPN to the backbone
self.bifpn = BiFPN(num_channels, num_layers)
# Define the rest of the YOLOv5 network
...
def forward(self, x):
# Backbone network forward pass
...
# Apply BiFPN
p3, p4, p5, p6, p7 = self.bifpn([p3, p4, p5, p6, p7])
# Rest of the YOLOv5 network forward pass
...
return outputs
```
这样,通过在YOLOv5的主干网络中添加BiFPN,可以增强模型对不同尺度目标的检测能力,提高检测精度和鲁棒性。
阅读全文
相关推荐
















