多项式输入C语言且能正确处理相应的系数与指数
时间: 2024-10-08 16:21:06 浏览: 47
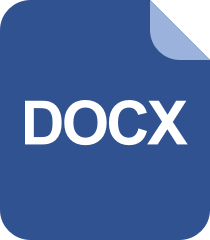
一元稀疏多项式计算器C语言课程设计.docx

在C语言中,为了正确处理多项式并存储其系数与指数,你可以创建一个自定义的数据结构,例如使用链表或者数组结合结构体来存储每个项。这里有一个基本的示例,使用结构体`PolynomialTerm`表示单个项(系数和指数),再用数组储存这些项:
```c
#include <stdio.h>
#include <stdlib.h> // 包含malloc和free函数
// 定义多项式项结构体
struct PolynomialTerm {
double coefficient; // 系数
int exponent; // 指数
};
// 定义多项式结构体
typedef struct {
size_t degree; // 当前最高次幂
struct PolynomialTerm terms[100]; // 可变大小的数组存放项
} Polynomial;
// 动态分配空间添加新的项
void add_term(Polynomial* poly, double coefficient, int exponent) {
if (poly->degree < sizeof(poly->terms)) {
poly->terms[poly->degree].coefficient = coefficient;
poly->terms[poly->degree].exponent = exponent;
poly->degree++;
} else {
// 如果已满,需要动态扩容
size_t new_size = poly->degree * 2; // 通常采用二倍增长策略
PolynomialTerm* new_terms = realloc(poly->terms, new_size * sizeof(PolynomialTerm));
if (!new_terms) { // 内存分配失败
perror("Memory allocation error");
exit(1);
}
poly->terms = new_terms;
poly->terms[poly->degree].coefficient = coefficient;
poly->terms[poly->degree].exponent = exponent;
poly->degree++;
}
}
// 评估多项式
double evaluate_polynomial(const Polynomial& poly, double x) {
double sum = 0.0;
for (size_t i = 0; i <= poly.degree; i++) {
sum += poly.terms[i].coefficient * pow(x, poly.terms[i].exponent);
}
return sum;
}
int main() {
Polynomial poly;
poly.degree = 0;
// 添加多项式项
add_term(&poly, 1.0, 2); // x^2
add_term(&poly, 3.0, 1); // 3x
add_term(&poly, 2.0, 0); // 2
// 输出多项式
printf("Polynomial: ");
for (size_t i = 0; i <= poly.degree; i++) {
printf("%.2fx^%d + ", poly.terms[i].coefficient, poly.terms[i].exponent);
}
printf("\n");
// 计算给定x的值
double x = 5.0;
double result = evaluate_polynomial(poly, x);
printf("Value at x = %.2lf: %.2lf\n", x, result);
return 0;
}
```
这个例子展示了如何创建一个可以动态添加和计算多项式值的基本结构。注意,这里的数组预设了最大100项,如果实际需要更多项,可以修改数组大小或使用更高效的数据结构。
阅读全文
相关推荐
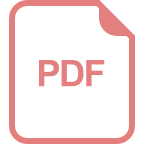
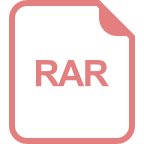
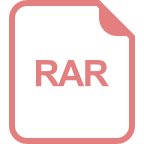
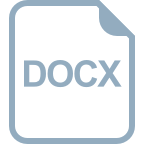
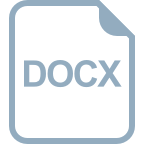
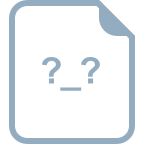
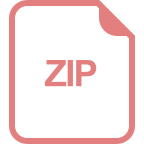
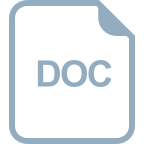
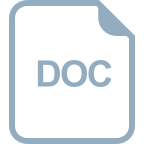
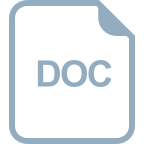
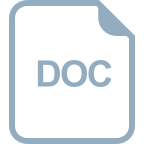
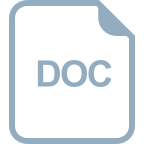
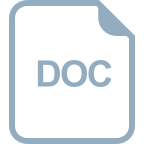
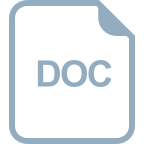
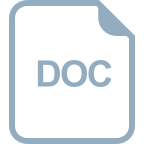
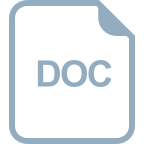
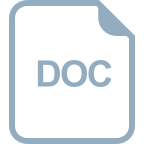
