c语言中四舍五入的函数
时间: 2024-12-15 15:13:35 浏览: 10
在C语言中,四舍五入通常通过数学库中的`<math.h>`头文件提供的函数来实现。这里有两个常用的函数:
1. `rint()` 或 `rintf()`: 这两个函数用于将双精度浮点数(`double`类型)四舍五入到最接近的整数。它们的区别在于:
- `rint(x)` 返回的是整型结果,适用于不需要精确小数部分的情况。
- `rintf(x)` 返回的是`float`类型的整数,保留浮点精度。
2. `round()` 或 `roundf()`: 这些函数同样用于将浮点数四舍五入,区别在于:
- `round(x)` 返回的是`long int`类型,如果`x`太大超过`int`范围,可能会返回非预期的结果。
- `roundf(x)` 类似于`rintf()`,处理浮点数并返回`float`类型结果。
使用这些函数时,一般会先包含`<math.h>`头文件,并确保处理好溢出或精度丢失的可能性。例如:
```c
#include <math.h>
double x = 3.14;
int rounded_int = rint(x);
float rounded_float = roundf(x);
```
相关问题
c语言浮点数四舍五入函数
在C语言中,可以使用库函数来实现浮点数的四舍五入操作。具体的函数为round(),它可以将一个浮点数四舍五入为最接近的整数。
使用该函数的原型为:
double round(double x)
需要注意的是,使用该函数前需要包含math.h头文件。
举个例子,假设有一个浮点数变量num,我们想要将它四舍五入为离它最接近的整数。可以使用如下代码:
#include <stdio.h>
#include <math.h>
int main() {
double num = 3.6;
double roundedNum = round(num);
printf("四舍五入后的数为:%f\n", roundedNum);
return 0;
}
输出结果为:四舍五入后的数为:4.000000
上述代码中,变量num的值为3.6。调用round()函数将其四舍五入为最接近的整数,将结果赋值给roundedNum变量。最后通过printf()函数将结果输出到屏幕上。
需要注意的是,round()函数的返回值仍然是一个浮点数,需要根据实际需求来确定是否需要将其转换为整数类型。另外,在某些情况下,四舍五入可能会存在一定的误差,这需要根据具体的需求来处理。
c语言float四舍五入函数
C语言并没有直接提供四舍五入到特定小数位的内置函数,但是你可以通过数学运算结合`<math.h>`库中的`round()`函数或者自定义算法来实现四舍五入。例如,如果你想将浮点数保留两位小数,可以这样做:
```c
#include <stdio.h>
#include <math.h>
// 自定义函数,四舍五入到指定小数位
float round_to_two_decimal_places(float num) {
float factor = 100; // 因为需要保留两位小数,所以乘以100
return round(num * factor) / factor;
}
int main() {
float num = 3.141592653589793;
float rounded_num = round_to_two_decimal_places(num);
printf("四舍五入后的结果: %.2f\n", rounded_num);
return 0;
}
```
在这个例子中,`round_to_two_decimal_places()`函数会先将输入的浮点数乘以100,然后对整数部分进行`round()`处理,最后除以100得到保留两位小数的结果。
阅读全文
相关推荐
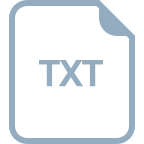
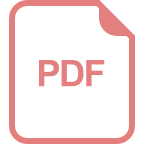













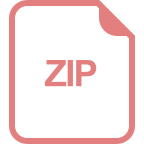
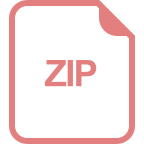