实现通过RSA进行加密和解密文本代码
时间: 2024-11-03 19:16:04 浏览: 20
RSA是一种非对称加密算法,它由Ron Rivest、Adi Shamir和Leonard Adleman在1977年发明。要使用RSA进行文本加密和解密,你需要进行以下几个步骤:
### 加密(公钥加密):
1. **生成密钥对**:首先生成一对公钥(`public_key`)和私钥(`private_key`)。公钥用于加密,私钥用于解密。
2. **接收者公开公钥**:发送方获取接收者的公钥。
3. **消息编码**:将明文转换成可以加密的形式,如Base64编码,以便与二进制数据兼容。
4. **加密过程**:使用接收者的公钥(即`e`(加密指数)和`n`(大素数))计算密文(`ciphertext`),公式通常是 `c = m^e mod n`,其中`m`是明文。
### 解密(私钥解密):
1. **接收密文**:接收者收到并保存来自发送方的加密信息。
2. **解密过程**:使用自己的私钥(即`d`(私钥指数)和`n`),通过公式 `m = c^d mod n` 计算出原始消息。
以下是使用Python实现RSA加密和解密的一个简化版示例(请注意这仅适用于演示,实际应用应考虑更安全的方式处理秘钥和错误处理):
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
import base64
def generate_keys():
key = RSA.generate(2048)
public_key = key.publickey().export_key('PEM')
private_key = key.export_key()
return public_key, private_key
def encrypt(message, recipient_public_key):
rsa_pubkey = RSA.import_key(base64.b64decode(recipient_public_key))
cipher_rsa = PKCS1_OAEP.new(rsa_pubkey)
ciphertext = cipher_rsa.encrypt(message.encode())
return base64.b64encode(ciphertext).decode()
def decrypt(ciphertext, sender_private_key):
rsa_privkey = RSA.import_key(base64.b64decode(sender_private_key))
cipher_rsa = PKCS1_OAEP.new(rsa_privkey)
plaintext = cipher_rsa.decrypt(base64.b64decode(ciphertext)).decode()
return plaintext
# 使用示例
public_key, private_key = generate_keys()
encrypted_message = encrypt("Hello, world!", public_key)
decrypted_message = decrypt(encrypted_message, private_key)
print(f"Encrypted message: {encrypted_message}")
print(f"Decrypted message: {decrypted_message}")
```
阅读全文
相关推荐
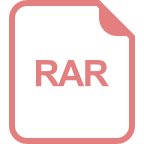
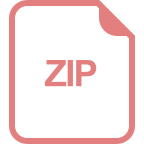
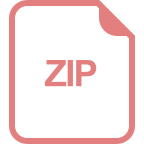



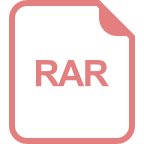
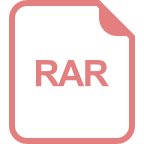
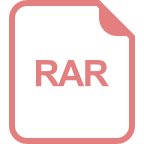
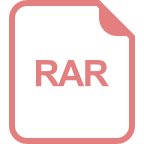
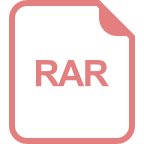
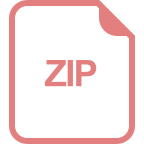
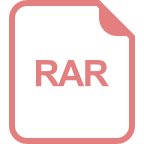
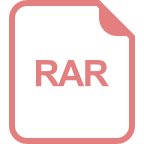
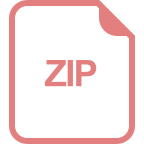
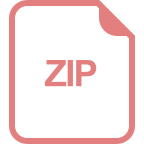
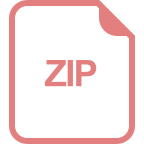
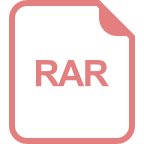
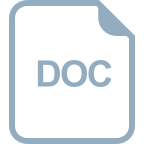