用C++实现通过RSA进行加密和解密文本;
时间: 2024-11-12 11:32:15 浏览: 27
在C++中,RSA(Rivest-Shamir-Adleman)是一种非对称加密算法,用于保护数据的安全传输。以下是基本步骤概述:
1. **生成公钥和私钥**:使用大素数p和q计算n=p*q,然后选择一个整数e(欧拉函数φ(n) = (p-1)*(q-1)的一个小素因子),并找到它的模逆d(满足 e * d ≡ 1 mod φ(n))。公钥是(e, n),私钥是(d, n)。
```cpp
#include <openssl/bio.h>
#include <openssl/rsa.h>
// Generate keys (private and public)
RSA* genKeys(int keyLength) {
RSA* rsa = RSA_new();
BIGNUM* p = BN_generate_prime(NULL, keyLength, NULL);
BIGNUM* q = BN_generate_prime(NULL, keyLength, NULL);
RSA_set0_factors(rsa, p, q); // Generate n = pq
RSA_generate_key_ex(rsa, keyLength, 65537, NULL, NULL); // Generate e
RSA_generate_key_ex(rsa, keyLength, d, NULL, NULL); // Generate d
BN_free(p);
BN_free(q);
return rsa;
}
```
2. **加密**:使用接收者的公钥(e, n)对明文进行加密,得到密文。
```cpp
std::string encrypt(const std::string& plaintext, const RSA* pubKey) {
BIO* bioIn = BIO_new_mem_buf(plaintext.c_str(), -1);
BIO* bioOut = BIO_new(BIO_s_mem());
int result = RSA_public_encrypt(strlen(plaintext), bioIn, bioOut, pubKey, RSA_PKCS1_OAEP_PADDING);
if (result <= 0) {
throw std::runtime_error("Encryption failed");
}
std::string ciphertext(bioOut->data(), bioOut->length());
BIO_free_all(bioIn);
BIO_free_all(bioOut);
return ciphertext;
}
```
3. **解密**:使用发送者的私钥(d, n)对密文进行解密,恢复原始信息。
```cpp
std::string decrypt(const std::string& ciphertext, const RSA* privKey) {
BIO* bioIn = BIO_new_mem_buf(ciphertext.c_str(), -1);
BIO* bioOut = BIO_new(BIO_s_mem());
int result = RSA_private_decrypt(strlen(ciphertext), bioIn, bioOut, privKey, RSA_PKCS1_OAEP_PADDING);
if (result <= 0) {
throw stdot::runtime_error("Decryption failed");
}
std::string plaintext(bioOut->data(), bioOut->length());
BIO_free_all(bioIn);
BIO_free_all(bioOut);
return plaintext;
}
```
注意:实际项目中需要处理错误检查、内存管理等问题,并且使用专业的库如 OpenSSL 进行操作,以上代码仅为示例。
阅读全文
相关推荐
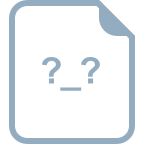
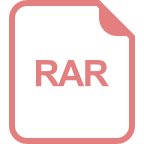
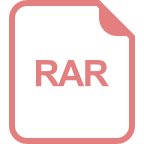
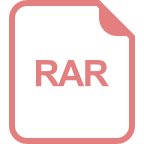
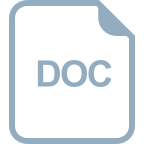
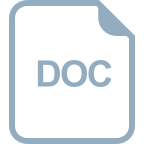
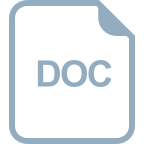



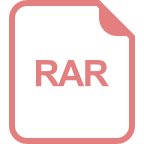
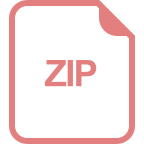
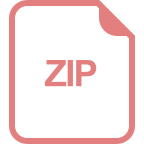
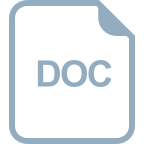
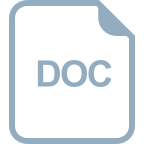
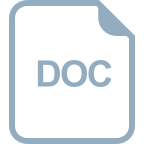
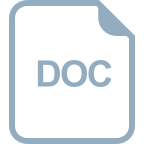
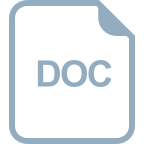
