编写一个c++程序,搜索int类型的数字文件,并将最大和最小的数字写入屏幕。该文件只包含int类型的数字,用空格或换行符分隔。如果这是作为班级作业完成的,请从您的教师那里获得文件名。
时间: 2024-09-27 11:15:34 浏览: 31
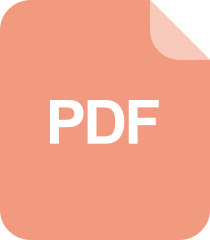
C++中进行txt文件读入和写入的方法示例

在C++中编写一个程序来搜索整数文件并找到最大和最小值,你可以按照以下步骤操作:
首先,你需要创建一个`ifstream`对象来读取文件。然后,定义两个变量分别存储当前找到的最大值和最小值,初始设置为整型的负无穷大和正无穷大。遍历文件中的每一行,通过`istringstream`解析每个数字,并更新最大值和最小值。
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <limits>
int main() {
// 获取文件名,这里假设用户输入或教师提供
std::string filename;
std::cout << "请输入文件名(例如: numbers.txt): ";
std::cin >> filename;
int max_value = INT_MIN;
int min_value = INT_MAX;
try {
std::ifstream file(filename);
if (!file) {
std::cerr << "无法打开文件: " << filename << std::endl;
return 1;
}
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
int num;
if (iss >> num) { // 检查是否成功读取整数
max_value = std::max(max_value, num);
min_value = std::min(min_value, num);
} else {
std::cerr << "无效的数字格式" << std::endl;
}
}
if (file)
file.close();
std::cout << "最大值: " << max_value << ", 最小值: " << min_value << std::endl;
} catch (const std::exception& e) {
std::cerr << "错误: " << e.what() << std::endl;
}
return 0;
}
```
阅读全文
相关推荐
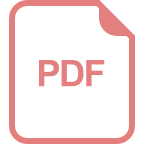
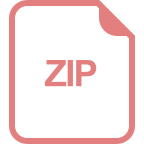
















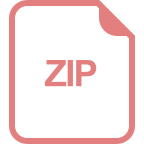