在words.txt文件中包含了87314个单词,编写c++程序从words文件中读取单词,并输出重复字母对最多的单词,将第一个最多重复字母对的单词写入newwords.txt文件中。例如tooth这
时间: 2023-04-26 22:02:02 浏览: 200
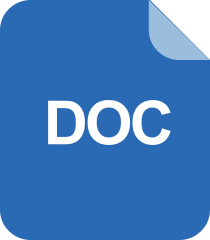
输入输出流-从words文件中读取单词,输出重复字母对最多的单词(C++)
个单词中,最多重复字母对为"oo",因此如果tooth是重复字母对最多的单词之一,则将其写入newwords.txt文件中。
回答:
这是一个需要读取文件并进行字符串处理的问题。可以使用C++的文件输入输出流来实现。
首先,需要打开words.txt文件并读取其中的单词。可以使用ifstream类来实现文件的读取操作。读取单词时,可以使用getline函数来读取一行字符串,并使用stringstream类将其分割成单词。
读取完所有单词后,需要对每个单词进行重复字母对的计数。可以使用一个循环来遍历每个单词,并使用一个map来记录每个单词中每个字母出现的次数。对于每个字母,可以使用一个嵌套循环来计算其与其他字母的重复次数。
最后,需要找到重复字母对最多的单词,并将其写入newwords.txt文件中。可以使用一个变量来记录当前最大的重复字母对数,并在遍历每个单词时更新该变量。如果当前单词的重复字母对数大于最大值,则将其写入newwords.txt文件中。
以下是一个可能的实现代码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <map>
#include <algorithm>
using namespace std;
int main() {
ifstream infile("words.txt");
ofstream outfile("newwords.txt");
if (!infile.is_open() || !outfile.is_open()) {
cout << "Error opening file!" << endl;
return 1;
}
string line, word;
map<char, int> freq;
int max_count = ;
while (getline(infile, line)) {
stringstream ss(line);
while (ss >> word) {
freq.clear();
int count = ;
for (int i = ; i < word.length(); i++) {
freq[word[i]]++;
for (int j = i + 1; j < word.length(); j++) {
if (word[i] == word[j]) {
count++;
}
}
}
if (count > max_count) {
max_count = count;
outfile << word << endl;
}
}
}
infile.close();
outfile.close();
return ;
}
```
阅读全文
相关推荐

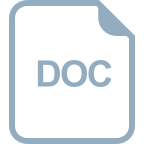













