Python中如何选择直线,然后在pyqt的GUI页面上绘制直线,并且设置绘制直线的颜色,以及可以计算绘制直线的长度
时间: 2024-11-29 20:34:56 浏览: 0
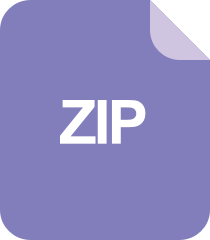
Python绘图画板程序 自由绘制 直线 矩形 椭圆 画笔调整 PyQt6
在Python的PyQt5中,你可以使用`PyQt5.QtWidgets`库创建GUI,并结合`PyQt5.QtGui`库的`QPainter`和`QGraphicsView`来进行交互式绘图。首先,你需要在图形界面中添加一个`QGraphicsView`作为绘图区域,然后通过鼠标事件处理来选择直线的起点和终点。
以下是一个简单的示例,演示如何选择两点并在直线上绘制颜色线段,并计算其长度:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QGraphicsScene, QGraphicsView, QGraphicsLineItem, QMenu
from PyQt5.QtGui import QPainter, QColor
from PyQt5.QtCore import Qt, QPointF
class GraphicsView(QWidget):
def __init__(self):
super(GraphicsView, self).__init__()
self.view = QGraphicsView()
self.scene = QGraphicsScene()
self.line_item = QGraphicsLineItem(QPointF(0, 0), QPointF(0, 0))
self.line_item.setPen(QColor('blue')) # 设置线条颜色为蓝色
self.scene.addItem(self.line_item)
self.view.setScene(self.scene)
self.line_length = 0
self.init_ui()
def init_ui(self):
self.setMouseTracking(True) # 允许鼠标跟踪
self.view.show()
def mouseMoveEvent(self, event): # 当鼠标移动时,更新线的位置
if event.buttons() & Qt.LeftButton:
line_start_point = self.line_item.pos()
new_point = QPointF(event.x(), event.y())
self.line_item.setLine(line_start_point, new_point)
self.update_line_length(new_point)
def update_line_length(self, end_point):
self.line_length = ((end_point - self.line_item.pos()).manhattanLength())
print(f"Line length: {self.line_length}")
if __name__ == '__main__':
app = QApplication(sys.argv)
main_widget = GraphicsView()
sys.exit(app.exec_())
```
在这个例子中,用户可以在画布上拖动鼠标选择直线的两个端点,线条的颜色默认为蓝色。每次移动鼠标都会更新线的位置,并计算出新线段的长度。
阅读全文
相关推荐
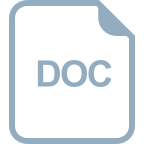
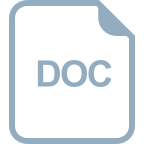


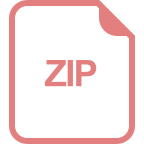
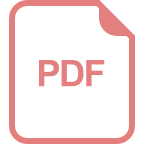
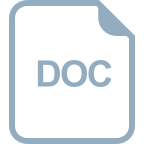
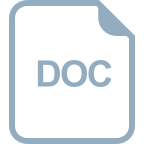









