数据结构c语言单列表的请输入节点,删除位置和逆置完整代码
时间: 2024-11-12 14:45:00 浏览: 3
在C语言中,我们通常会创建链表数据结构来实现单向列表。以下是创建一个简单的单链表节点、插入节点、删除节点以及逆置链表的基本代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 节点值
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点
Node* create_node(int value) {
Node* new_node = (Node*)malloc(sizeof(Node));
if (new_node) {
new_node->data = value;
new_node->next = NULL;
}
return new_node;
}
// 在指定位置插入节点
void insert_at_position(Node** head, int value, int position) {
if (*head == NULL || position <= 0) return; // 如果链表为空或位置无效
Node* newNode = create_node(value);
if (position == 1) { // 插入到头节点
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int i = 1; i < position - 1 && current != NULL; ++i) {
current = current->next;
}
if (current != NULL) { // 找到了有效位置
newNode->next = current->next;
current->next = newNode;
}
}
}
// 删除指定位置的节点
void delete_node(Node** head, int position) {
if (*head == NULL || position <= 0) return;
if (position == 1) { // 删除头节点
Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
Node* current = *head;
for (int i = 1; i < position - 1 && current != NULL; ++i) {
current = current->next;
}
if (current != NULL) { // 找到了有效位置
Node* temp = current->next;
current->next = temp->next;
free(temp);
}
}
}
// 逆置链表
void reverse_list(Node** head) {
Node* prev = NULL;
Node* current = *head;
Node* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*head = prev;
}
// 遍历并打印链表
void print_list(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
// 插入一些节点示例
insert_at_position(&head, 1, 1); // [1]
insert_at_position(&head, 2, 2); // [1, 2]
insert_at_position(&head, 3, 3); // [1, 2, 3]
printf("Original list: ");
print_list(head);
// 删除节点示例
delete_node(&head, 2); // [1, 3]
printf("\nList after deletion: ");
print_list(head);
// 逆置链表
reverse_list(&head);
printf("\nReversed list: ");
print_list(head);
return 0;
}
```
阅读全文
相关推荐
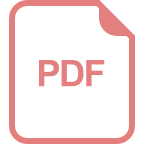
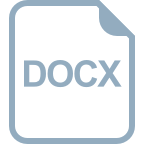
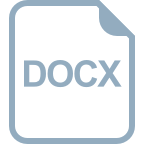


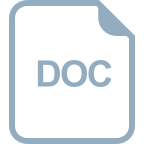
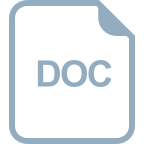
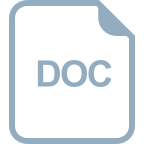





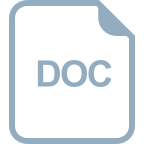
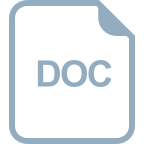
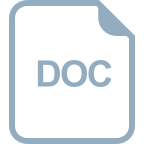

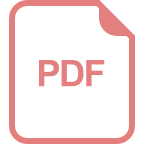
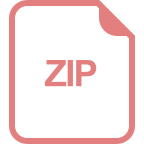