python实现AES算法的文件加密代码准确
时间: 2024-09-30 15:09:29 浏览: 40
在Python中,我们可以使用`cryptography`库来实现AES(高级加密标准)算法的文件加密。以下是一个简单的示例,展示了如何使用AES对文件进行加密和解密:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from cryptography.hazmat.backends import default_backend
import os
def generate_key(password, salt, iterations):
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32,
salt=salt,
iterations=iterations,
backend=default_backend()
)
key = kdf.derive(password.encode())
return key
def encrypt_file(file_path, output_path, password):
with open(file_path, 'rb') as in_file:
data = in_file.read()
salt = os.urandom(16)
key = generate_key(password, salt, 100000) # 强度较高的迭代次数
cipher = Cipher(algorithms.AES(key), modes.CBC(os.urandom(16)), backend=default_backend())
encryptor = cipher.encryptor()
ct_bytes = encryptor.update(data) + encryptor.finalize()
iv = cipher.iv
encrypted_data = iv + ct_bytes
with open(output_path, 'wb') as out_file:
out_file.write(encrypted_data)
def decrypt_file(input_path, output_path, password):
with open(input_path, 'rb') as in_file:
encrypted_data = in_file.read()
iv = encrypted_data[:16]
ct_bytes = encrypted_data[16:]
key = generate_key(password, iv, 100000) # 使用相同的盐和迭代次数
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=default_backend())
decryptor = cipher.decryptor()
pt_bytes = decryptor.update(ct_bytes) + decryptor.finalize()
with open(output_path, 'wb') as out_file:
out_file.write(pt_bytes)
# 示例用法
password = "your_password"
encrypt_file("input.txt", "output.enc", password)
decrypt_file("output.enc", "output_decrypted.txt", password)
阅读全文
相关推荐
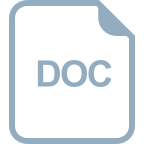
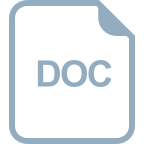
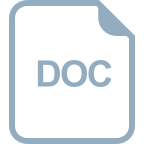




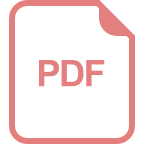
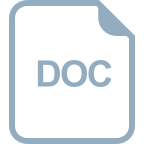
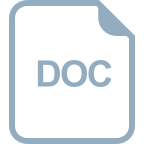








