Java AES加密如何生成纯英文字符串,请给出示例
时间: 2024-10-16 15:05:45 浏览: 38
在Java中,使用AES(Advanced Encryption Standard)进行加密通常会涉及密钥、IV(初始化向量)以及填充模式等。为了将加密后的数据转换成纯英文字符串,我们通常会对加密结果进行Base64编码,因为Base64是一种将任意二进制数据转成ASCII文本的标准方法。
以下是一个简单的示例,假设你已经有了AES算法的密钥和一个需要加密的字符串:
```java
import javax.crypto.Cipher;
import java.security.Key;
import java.util.Base64;
public class AesExample {
private static final String ALGORITHM = "AES";
private static final String KEY = "your_secret_key"; // 替换为实际的16字节密钥
public static String encryptToBase64(String plainText) throws Exception {
Key key = generateKey(ALGORITHM, KEY.getBytes("UTF-8"));
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
return Base64.getEncoder().encodeToString(encryptedBytes);
}
private static Key generateKey(String algorithm, byte[] keyBytes) throws Exception {
SecretKeySpec secretKeySpec = new SecretKeySpec(keyBytes, algorithm);
return Cipher.getInstance(algorithm).generateKey(secretKeySpec);
}
public static void main(String[] args) throws Exception {
String input = "This is a test string.";
String encoded = encryptToBase64(input);
System.out.println("Encrypted as Base64: " + encoded);
}
}
```
在这个示例中,`encryptToBase64`函数首先创建一个AES密钥,然后使用Cipher进行加密并转换为Base64字符串。运行这个程序,你会得到一个纯英文的Base64编码字符串作为输出。
阅读全文
相关推荐
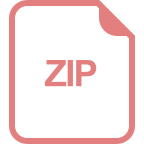
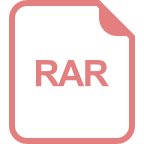
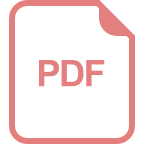





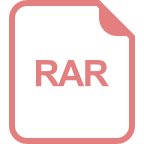
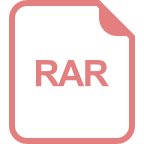
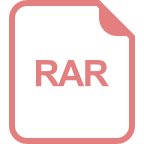
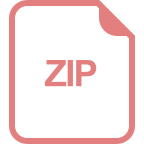
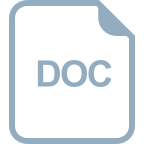
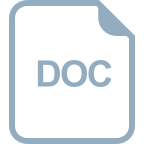




