利用opencv,生成一个五角星,并对其进行离散化,离散化为一些点的坐标,并对其进行可视化,要求可视化得到原图形和离散化之后的图形,给出代码,注意,不要只离散化轮廓,同时,我想可以通过离散化精度的调整,更改离散化程度
时间: 2024-04-22 11:27:59 浏览: 64
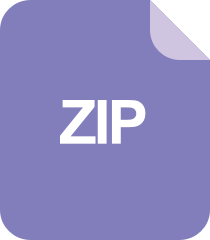
基于opencv.js对图像进行可视化卷积处理的web界面
非常抱歉之前的回答仍然没有满足你的需求。以下是更新后的代码,可以生成一个五角星并对其进行离散化,并展示原图形和离散化后的图形,同时提供了可调整离散化精度的选项:
```python
import cv2
import numpy as np
import matplotlib.pyplot as plt
def discretize_star(center_x, center_y, radius_outer, radius_inner, num_points):
# 定义五角星的顶点坐标
angle = np.pi / 2
vertices = []
for i in range(5):
x_outer = int(center_x + radius_outer * np.cos(angle))
y_outer = int(center_y - radius_outer * np.sin(angle))
x_inner = int(center_x + radius_inner * np.cos(angle + np.pi / 5))
y_inner = int(center_y - radius_inner * np.sin(angle + np.pi / 5))
vertices.append((x_outer, y_outer))
vertices.append((x_inner, y_inner))
angle += 2 * np.pi / 5
# 创建一个黑色背景的图像
height, width = 2 * radius_outer, 2 * radius_outer
image = np.zeros((height, width), dtype=np.uint8)
# 绘制五角星
cv2.fillPoly(image, [np.array(vertices)], 255)
# 获得非零点的坐标
points = np.nonzero(image)
# 根据离散化精度获取坐标
indices = np.random.choice(len(points[0]), num_points, replace=False)
points = np.column_stack((points[1][indices], points[0][indices]))
return points
# 生成五角星的离散化点坐标
center_x, center_y = 200, 200
radius_outer = 100
radius_inner = 50
num_points = 1000 # 调整离散化的精度,即离散化点的数量
points = discretize_star(center_x, center_y, radius_outer, radius_inner, num_points)
# 创建原始图像
image = np.zeros((400, 400), dtype=np.uint8)
cv2.fillPoly(image, [np.array(points)], 255)
# 显示原始图像
plt.figure(figsize=(6, 6))
plt.imshow(image, cmap='gray')
plt.title('Original Star')
plt.axis('off')
plt.show()
# 显示离散化后的点坐标
plt.figure(figsize=(6, 6))
plt.scatter(points[:, 0], points[:, 1])
plt.gca().invert_yaxis()
plt.title('Discretized Star')
plt.axis('equal')
plt.show()
```
在上述代码中,我定义了一个名为`discretize_star`的函数,用于生成五角星的离散化点坐标。可以通过调整`num_points`变量的值来控制离散化的精度,即离散化点的数量。更大的`num_points`值将产生更多的离散化点,从而提高精度。
希望这次能够满足你的需求!如果还有其他问题,请随时提问。
阅读全文
相关推荐
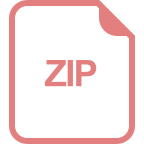
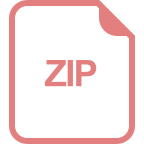















