GAT pytorch
时间: 2023-10-29 16:53:17 浏览: 54
GAT(Graph Attention Network)是一种用于图数据的注意力机制模型,在PyTorch中也有相应的实现。您可以使用PyTorch Geometric库来构建和训练GAT模型。
要使用GAT模型,您首先需要安装PyTorch Geometric库。您可以使用以下命令安装它:
```
pip install torch-scatter -f https://pytorch-geometric.com/whl/torch-1.9.0+${CUDA}.html
pip install torch-sparse -f https://pytorch-geometric.com/whl/torch-1.9.0+${CUDA}.html
pip install torch-cluster -f https://pytorch-geometric.com/whl/torch-1.9.0+${CUDA}.html
pip install torch-spline-conv -f https://pytorch-geometric.com/whl/torch-1.9.0+${CUDA}.html
pip install torch-geometric
```
一旦安装完成,您可以使用以下代码示例构建和训练一个简单的GAT模型:
```python
import torch
import torch.nn.functional as F
from torch_geometric.nn import GATConv
class GAT(torch.nn.Module):
def __init__(self, input_dim, hidden_dim, num_classes):
super(GAT, self).__init__()
self.conv1 = GATConv(input_dim, hidden_dim, heads=8)
self.conv2 = GATConv(hidden_dim * 8, hidden_dim, heads=1)
self.fc = torch.nn.Linear(hidden_dim, num_classes)
def forward(self, x, edge_index):
x = F.dropout(x, p=0.6, training=self.training)
x = F.elu(self.conv1(x, edge_index))
x = F.dropout(x, p=0.6, training=self.training)
x = self.conv2(x, edge_index)
x = F.dropout(x, p=0.6, training=self.training)
x = F.elu(self.fc(x))
return F.log_softmax(x, dim=1)
# 构造数据
x = torch.randn(10, 16) # 节点特征矩阵
edge_index = torch.tensor([[0, 1, 1, 2, 3, 4, 5, 6, 8, 7],
[1, 0, 2, 1, 4, 3, 6, 5, 7, 8]], dtype=torch.long) # 边索引
y = torch.tensor([0, 1, 0, 1, 0, 1, 0, 1, 0, 1], dtype=torch.long) # 节点标签
# 初始化模型并进行训练
model = GAT(input_dim=16, hidden_dim=32, num_classes=2)
optimizer = torch.optim.Adam(model.parameters(), lr=0.01)
def train():
model.train()
optimizer.zero_grad()
out = model(x, edge_index)
loss = F.nll_loss(out, y)
loss.backward()
optimizer.step()
for epoch in range(100):
train()
```
这个示例代码构建了一个包含两个GAT层的GAT模型,并在一个简单的图数据集上进行了训练。您可以根据自己的数据集和任务来调整模型的参数和配置。请确保根据您的需求适当调整模型的输入维度、隐藏维度和输出类别数量等参数。
相关推荐
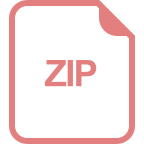
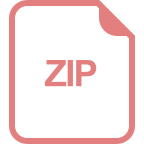














