基于pytorch的gat实现 python
时间: 2024-01-09 22:01:48 浏览: 106
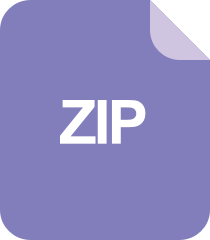
python实现神经网络,从入门到精通,CNN卷积神经网络,循环神经网络网络 使用pytorch库实现
GAT(Graph Attention Network)是一种基于图神经网络的模型,是一种用于图数据的深度学习模型。下面是一个基于PyTorch实现GAT模型的Python代码示例:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class GraphAttentionLayer(nn.Module):
def __init__(self, in_features, out_features):
super(GraphAttentionLayer, self).__init__()
self.W = nn.Linear(in_features, out_features)
self.a = nn.Linear(2 * out_features, 1)
def forward(self, h, adj):
Wh = self.W(h)
a_input = self.prepare_attention_input(Wh)
e = F.leaky_relu(self.a(a_input), negative_slope=0.2)
attention = F.softmax(e, dim=1)
h_prime = self.aggregate_neighborhood(Wh, adj, attention)
return h_prime
def prepare_attention_input(self, h):
N = h.size()[0]
h_repeat = h.repeat(N, 1)
h_repeat_reverse = h_repeat[::-1]
return torch.cat([h_repeat_reverse, h_repeat], dim=1)
def aggregate_neighborhood(self, Wh, adj, attention):
Wh_repeat = Wh.repeat(1, adj.size()[0]).view(adj.size()[0] * adj.size()[0], -1)
attention_flat = attention.view(-1)
neighborhood = attention_flat * Wh_repeat
h_prime = torch.matmul(adj, neighborhood)
return h_prime
class GAT(nn.Module):
def __init__(self, num_features, num_classes, num_heads, hidden_units):
super(GAT, self).__init__()
self.attentions = nn.ModuleList()
for _ in range(num_heads):
self.attentions.append(GraphAttentionLayer(num_features, hidden_units))
self.out_att = GraphAttentionLayer(hidden_units * num_heads, num_classes)
def forward(self, x, adj):
GAT_outputs = [attention(x, adj) for attention in self.attentions]
x = torch.cat(GAT_outputs, dim=1)
x = F.dropout(x, p=0.5, training=self.training)
x = self.out_att(x, adj)
return F.log_softmax(x, dim=1)
```
这段代码实现了一个包含多个头注意力机制的GAT模型。`GraphAttentionLayer`类定义了一个图注意力层的操作,而`GAT`类则是将多个注意力层组合在一起,构成了一个完整的GAT模型。在`forward`函数中,输入数据经过注意力层以及激活函数的处理后,输出最终的类别概率分布。
以上就是基于PyTorch实现的GAT模型的Python代码示例。希望对你有所帮助!
阅读全文
相关推荐
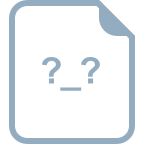
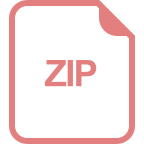



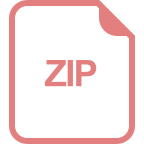
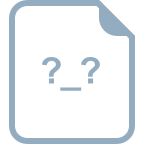
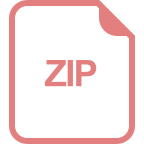
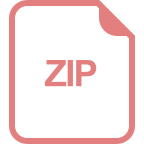
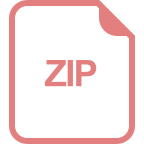
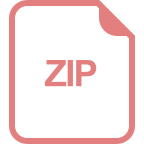
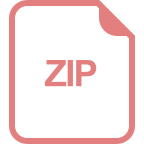
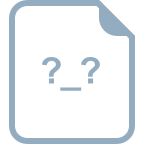



