用C语言编写一个用于统计灰度级频数和灰度级频率直方图的程序?
时间: 2024-10-06 18:05:57 浏览: 35
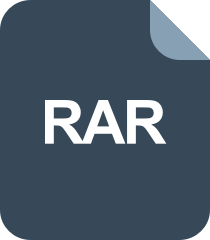
应用MATLAB(或C)语言编写一幅灰度图像直方图统计程序

在C语言中,你可以使用数组来创建一个简单的灰度级频率直方图。下面是一个基本的步骤描述:
首先,你需要定义一个足够大的数组,数组的长度是你想要统计的最大灰度级别数加一(通常8位图像的灰度级别是0-255)。然后读取图像数据,对于每个像素,将对应的灰度值作为数组下标的索引来增加计数值。
```c
#include <stdio.h>
#define MAX_GRAY_LEVEL 256
int histogram[MAX_GRAY_LEVEL];
void count_gray_levels(unsigned char *image_data, int width, int height) {
for (int i = 0; i < width * height; ++i) {
histogram[image_data[i]]++;
}
}
// 打印直方图
void print_histogram() {
for (int i = 0; i < MAX_GRAY_LEVEL; ++i) {
if (histogram[i] > 0) {
printf("%d: %d\n", i, histogram[i]);
} else {
printf("%d: %d\n", i, 0); // 如果某个级别未出现,显示0
}
}
}
int main(int argc, char *argv[]) {
// 从文件加载图像数据并计算直方图
unsigned char *image_data = load_image_from_file(argv[1]); // 假设有一个load_image_from_file函数来读取图像
int width = ...; // 图像宽度
int height = ...; // 图像高度
count_gray_levels(image_data, width, height);
print_histogram();
return 0;
}
```
注意,这个示例假设你已经有了`load_image_from_file`函数来处理图像的输入,实际项目中需要你自己实现这个功能。同时,为了简洁,这里没有包含错误处理和内存管理的部分。
阅读全文
相关推荐
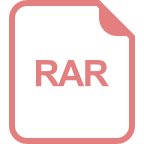
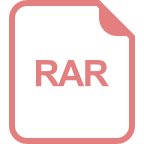















