python爬虫爬取气温数据
时间: 2024-12-25 14:12:19 浏览: 4
Python爬虫可以用来抓取网页上的气温数据,通常涉及到API接口访问或HTML页面解析。如果你的目标是获取实时天气信息,很多天气服务提供API,如中国气象局的API、OpenWeatherMap等,你可以通过发送HTTP请求并处理返回的JSON或XML数据来获取气温。
例如,使用requests库发送GET请求到提供气温数据的API,并使用json或xml库解析响应:
```python
import requests
def fetch_weather_data(city):
api_url = "http://api.weather.com/weather?city=" + city + "&key=your_api_key"
response = requests.get(api_url)
if response.status_code == 200:
data = response.json() # 如果是JSON格式
temperature = data["temperature"]
return temperature
else:
print("Failed to fetch data")
# 使用示例
temperature = fetch_weather_data("北京")
print(f"当前北京的气温是 {temperature} 度.")
相关问题
python爬虫爬取年际气温数据
### 使用 Python 编写爬虫程序抓取年度气温数据
为了实现这一目标,可以采用 `requests` 和 `BeautifulSoup` 库来处理网页请求与解析 HTML 文档。对于结构化较好的页面,如表格形式展示的数据,还可以考虑使用 Pandas 的 `read_html()` 方法简化操作过程[^3]。
#### 准备工作
安装必要的第三方库:
```bash
pip install requests beautifulsoup4 pandas lxml openpyxl
```
#### 获取并分析目标网页内容
通过发送 HTTP 请求访问指定 URL 地址获取所需年份的温度资料:
```python
import requests
from bs4 import BeautifulSoup as BSoup
import pandas as pd
def fetch_temperature_data(year):
url = f"https://www.example.com/weather/{year}" # 替换为目标站点的实际链接
response = requests.get(url)
if not response.ok:
raise Exception(f"Failed to load page {url}")
soup = BSoup(response.text, 'lxml')
table = soup.find('table', {'class': 'weather-data'}) # 查找特定样式类别的表格元素
df_list = pd.read_html(str(table))
temperature_df = df_list[0]
return temperature_df
```
此函数接收一个参数 year 表示想要查询的具体年份,并返回包含当月平均最高温最低温和降水量等信息的数据框对象。
#### 数据清洗与保存至 Excel 文件
对原始数据进行初步清理去除不必要的列或行之后再导出到本地磁盘上便于后续可视化或其他用途:
```python
def clean_and_save(df, filename="temperature.xlsx"):
cleaned_df = (
df.dropna(how='all') # 删除完全为空白的所有行
.drop(columns=['Unnamed:*']) # 移除未命名字段
[['Date', 'Max Temp (°C)', ...]] # 只保留日期以及感兴趣的几项指标
)
writer = pd.ExcelWriter(filename, engine='openpyxl')
cleaned_df.to_excel(writer, index=False)
writer.save()
```
最后调用上述定义好的两个辅助方法完成整个流程即可获得一份完整的年度气象统计报表。
python爬虫爬取全年天气数据代码
Python 爬虫用于获取网络数据,例如天气数据,通常需要结合一些库如 requests 和 BeautifulSoup 或者更现代的 Scrapy 框架。以下是一个基本的 Python 爬取全年天气数据的简单示例,假设我们要从一个提供 API 的网站获取天气信息:
```python
import requests
import json
# 假设 API 地址和所需的查询参数
api_url = "http://api.weather.com/weather/v1/place/{city}?apiKey={your_api_key}"
city_name = "北京"
params = {
'location': city_name,
'timeframe': 'year', # 获取全年的数据
}
def get_weather_data():
response = requests.get(api_url.format(city=city_name), params=params)
if response.status_code == 200:
data = json.loads(response.text) # 解析 JSON 格式的数据
weather_data = [day['data'] for day in data['dailyForecasts']] # 提取每日天气信息
return weather_data
else:
print(f"请求失败,状态码: {response.status_code}")
return None
# 使用获取到的数据
weather_data = get_weather_data()
if weather_data:
# 对数据进行进一步处理,例如存储到文件或数据库
for day_data in weather_data:
# 打印每一天的气温等信息
print(day_data['temperatureMax'], day_data['temperatureMin'])
else:
print("无法获取数据")
阅读全文
相关推荐
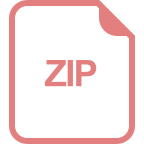
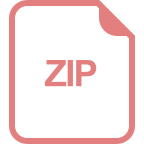
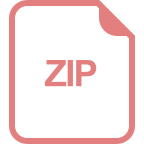



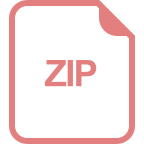
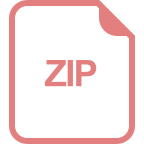
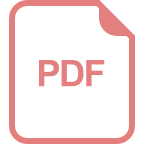
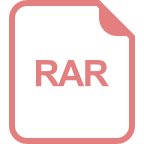
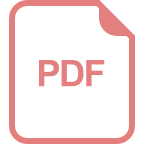
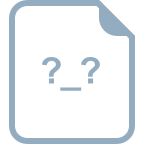
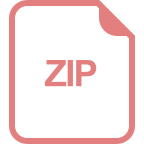
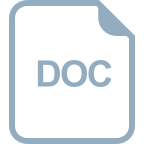


