如何用编程语言(如C++或Python)实现一个名为`locateVex`的数据结构函数,用于操作图的数据结构?这个函数应该能够找到特定顶点在图中的位置或者表示其在邻接列表或邻接矩阵中的索引,请提供相关的代码实现和解释说明。
时间: 2024-11-12 09:36:16 浏览: 72
在图数据结构的实现中,`locateVex`函数并不直接对应于一个特定的数据结构,而是描述了一个操作,即查找给定顶点在图中的表示。在邻接列表或邻接矩阵这两种常见的图表示法中,每个顶点通常有一个唯一的标识符,如整数ID。以下是一个简化的例子,分别展示了如何在C++和Python中使用邻接列表来实现这个函数:
**C++(邻接列表)**
```cpp
#include <unordered_map>
// 定义一个简单的邻接列表类
class Graph {
public:
struct Node {
int vertex;
std::vector<int> neighbors; // 邻接节点列表
};
void addVertex(int id) { vertices[id] = Node{id}; }
int locateVex(int vertexId) {
auto it = vertices.find(vertexId);
if (it != vertices.end()) {
return it->second.vertex;
}
throw std::runtime_error("Vertex not found");
}
private:
std::unordered_map<int, Node> vertices;
};
```
在这个例子中,`addVertex`用于添加新顶点,并使用顶点ID作为键值对的键。`locateVex`接受一个顶点ID,如果找到,则返回它的索引;找不到则抛出异常。
**Python(邻接列表)**
```python
class Graph:
def __init__(self):
self.vertices = {} # 使用字典表示邻接列表
def add_vertex(self, vertex_id):
self.vertices[vertex_id] = []
def locate_vex(self, vertex_id):
if vertex_id in self.vertices:
return vertex_id # 返回顶点ID本身作为索引
else:
raise ValueError("Vertex not found")
# 示例用法
g = Graph()
g.add_vertex(0)
print(g.locate_vex(0)) # 输出: 0
```
这两个示例都假设了图的每个顶点都有一个独特的ID,这是`locateVex`函数的基础。实际的实现可能会根据需求更复杂,比如图可能存储边的信息而不是仅仅顶点连接关系。
阅读全文
相关推荐



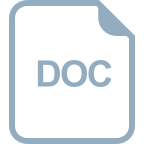













