如何在c语言中建立链表
时间: 2024-10-09 20:04:21 浏览: 27
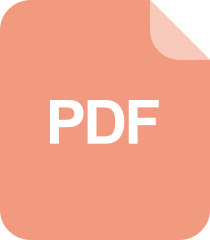
C语言如何建立链表并实现增删查改详解
在 C 语言中建立链表通常需要定义链表节点结构、创建节点、以及连接节点等步骤。这里提供一个简单的例子来说明如何实现一个单向动态链表。
**1. 定义链表节点结构**:
```c
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
**2. 创建节点函数**:
```c
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
if(newNode == NULL) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL; // 初始化新节点的 next 指针为空
return newNode;
}
```
**3. 连接链表**:
```c
void appendToList(Node** head, int value) {
if(*head == NULL) { // 链表为空,直接创建新节点
*head = createNode(value);
} else {
Node* lastNode = *head;
while(lastNode->next != NULL) {
lastNode = lastNode->next;
}
lastNode->next = createNode(value); // 将新节点添加到链表末尾
}
}
```
**4. 打印链表**:
```c
void printList(Node* head) {
Node* currentNode = head;
while(currentNode != NULL) {
printf("%d ", currentNode->data);
currentNode = currentNode->next;
}
printf("\n");
}
```
**完整示例**:
```c
int main() {
Node* head = NULL;
appendToList(&head, 1);
appendToList(&head, 2);
appendToList(&head, 3);
printList(head); // 输出: 1 2 3
return 0;
}
```
在这个例子中,`appendToList`函数用于在链表末尾添加新节点,`createNode`负责创建新节点,`printList`则用于遍历链表并打印节点值。
阅读全文
相关推荐
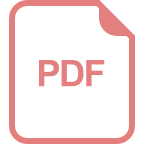
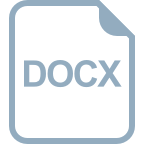
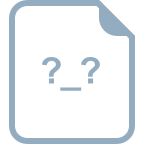

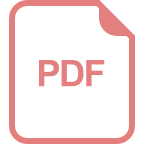
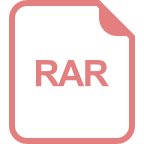
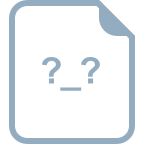
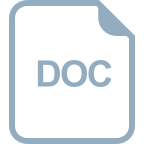








