请帮我用java代码写一个德州扑克牌型判断
时间: 2024-04-13 13:29:11 浏览: 25
当然,以下是一个简单的Java代码示例,用于判断德州扑克的牌型:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class PokerHandEvaluator {
public static void main(String[] args) {
List<Card> hand = new ArrayList<>();
hand.add(new Card("Spades", "A"));
hand.add(new Card("Hearts", "K"));
hand.add(new Card("Diamonds", "Q"));
hand.add(new Card("Spades", "J"));
hand.add(new Card("Spades", "10"));
PokerHandType handType = evaluateHand(hand);
System.out.println("Hand Type: " + handType);
}
public static PokerHandType evaluateHand(List<Card> hand) {
Collections.sort(hand);
if (isRoyalFlush(hand)) {
return PokerHandType.ROYAL_FLUSH;
}
if (isStraightFlush(hand)) {
return PokerHandType.STRAIGHT_FLUSH;
}
if (isFourOfAKind(hand)) {
return PokerHandType.FOUR_OF_A_KIND;
}
// Check for other hand types...
return PokerHandType.HIGH_CARD;
}
// Check if the hand is a Royal Flush (A, K, Q, J, 10 of the same suit)
public static boolean isRoyalFlush(List<Card> hand) {
return isStraightFlush(hand) && hand.get(0).getValue().equals("A");
}
// Check if the hand is a Straight Flush (five consecutive cards of the same suit)
public static boolean isStraightFlush(List<Card> hand) {
return isStraight(hand) && isFlush(hand);
}
// Check if the hand is a Four of a Kind (four cards of the same rank)
public static boolean isFourOfAKind(List<Card> hand) {
for (int i = 0; i <= hand.size() - 4; i++) {
if (hand.get(i).getValue().equals(hand.get(i + 3).getValue())) {
return true;
}
}
return false;
}
// Check if the hand is a Straight (five consecutive cards)
public static boolean isStraight(List<Card> hand) {
for (int i = 0; i < hand.size() - 1; i++) {
if (hand.get(i).getRank() != hand.get(i + 1).getRank() - 1) {
return false;
}
}
return true;
}
// Check if the hand is a Flush (all cards of the same suit)
public static boolean isFlush(List<Card> hand) {
String suit = hand.get(0).getSuit();
for (int i = 1; i < hand.size(); i++) {
if (!hand.get(i).getSuit().equals(suit)) {
return false;
}
}
return true;
}
}
class Card implements Comparable<Card> {
private String suit;
private String value;
public Card(String suit, String value) {
this.suit = suit;
this.value = value;
}
public String getSuit() {
return suit;
}
public String getValue() {
return value;
}
public int getRank() {
if (value.equals("A")) {
return 14; // Ace is the highest rank
} else if (value.equals("K")) {
return 13;
} else if (value.equals("Q")) {
return 12;
} else if (value.equals("J")) {
return 11;
} else if (value.equals("10")) {
return 10;
} else {
return Integer.parseInt(value);
}
}
@Override
public int compareTo(Card other) {
return Integer.compare(this.getRank(), other.getRank());
}
}
enum PokerHandType {
ROYAL_FLUSH,
STRAIGHT_FLUSH,
FOUR_OF_A_KIND,
// Add other hand types as needed...
HIGH_CARD
}
```
这段代码实现了一个简单的德州扑克牌型判断。你可以根据需要在`evaluateHand`方法中添加其他牌型的判断逻辑,或者修改`Card`类中的牌面值和花色,以及`PokerHandType`枚举类中的牌型。
相关推荐
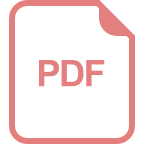
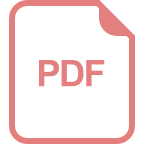
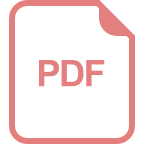














