java实现广度优先遍历
时间: 2024-06-18 18:02:50 浏览: 26
Java实现广度优先遍历可以使用队列来实现。具体步骤如下:
1. 将起始节点放入队列中
2. 取出队首节点,将其未访问过的相邻节点全部放入队列中
3. 标记当前节点为已访问
4. 重复步骤2和3直到队列为空
以下是Java代码实现广度优先遍历的示例:
```
import java.util.*;
public class BFS {
public static void bfs(Node node) {
Queue<Node> queue = new LinkedList<>();
queue.offer(node);
node.visited = true;
while (!queue.isEmpty()) {
Node current = queue.poll();
System.out.println(current.value);
for (Node neighbor : current.neighbors) {
if (!neighbor.visited) {
queue.offer(neighbor);
neighbor.visited = true;
}
}
}
}
static class Node {
int value;
List<Node> neighbors;
boolean visited;
public Node(int value) {
this.value = value;
this.neighbors = new ArrayList<>();
this.visited = false;
}
}
public static void main(String[] args) {
Node node1 = new Node(1);
Node node2 = new Node(2);
Node node3 = new Node(3);
Node node4 = new Node(4);
Node node5 = new Node(5);
node1.neighbors.add(node2);
node1.neighbors.add(node3);
node2.neighbors.add(node4);
node3.neighbors.add(node4);
node3.neighbors.add(node5);
bfs(node1);
}
}
```
相关推荐
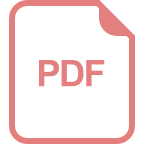
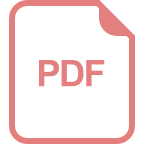
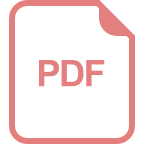
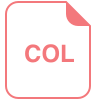
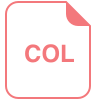
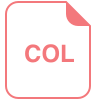
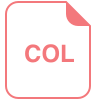
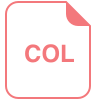









