本关任务:有三个候选人,每个选民只能投一票,写一程序,用结构体存储数据,求出得票结果。 三个候选人为 "Li", "Zhang","Sun"。
时间: 2024-09-12 13:06:27 浏览: 164
要编写一个程序来计算三位候选人 "Li"、"Zhang" 和 "Sun" 的得票结果,我们可以创建一个结构体来存储每个候选人的名字和对应的投票数。首先,定义一个结构体 `Candidate`:
```c++
#include <string>
#include <map>
struct Candidate {
std::string name;
int votes;
// 默认构造函数和用于比较名称的默认析构函数
Candidate(const std::string& n = "") : name(n), votes(0) {}
bool operator<(const Candidate& other) const { return name < other.name; }
};
```
接下来,创建一个 map 来存储候选人的名字和投票计数,初始化并允许用户输入投票:
```c++
int main() {
std::map<Candidate, int> electionResults;
// 初始化候选人
Candidate li("Li");
Candidate zhang("Zhang");
Candidate sun("Sun");
electionResults[li] = 0;
electionResults[zhang] = 0;
electionResults[sun] = 0;
// 接收用户投票
int totalVotes;
std::cout << "Enter the total number of votes: ";
std::cin >> totalVotes;
for (int i = 0; i < totalVotes; ++i) {
std::string voterChoice;
std::cout << "Enter vote for Li, Zhang or Sun (case-sensitive): ";
std::cin >> voterChoice;
if (voterChoice == "Li") {
electionResults[li]++;
} else if (voterChoice == "Zhang") {
electionResults[zhang]++;
} else if (voterChoice == "Sun") {
electionResults[sun]++;
} else {
std::cerr << "Invalid choice! Please try again.\n";
continue;
}
}
// 打印得票结果
for (const auto& result : electionResults) {
std::cout << result.first.name << " received " << result.second << " votes.\n";
}
return 0;
}
```
阅读全文
相关推荐
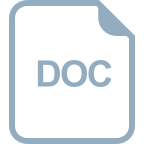
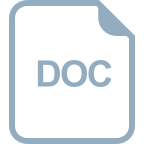
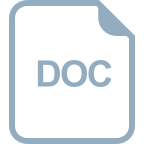















