生成一个完整的:DH秘钥交换实现 Java代码
时间: 2024-11-11 07:34:10 浏览: 22
Diffie-Hellman(DH)密钥交换是一种非对称加密技术,用于两个通信方在不共享预先秘密的情况下建立共享密钥。以下是一个简单的Java实现,使用`java.math.BigInteger`和`javax.crypto.interfaces.DHParameterSpec`:
```java
import javax.crypto.spec.DHParameterSpec;
import java.math.BigInteger;
public class DHKeyExchangeExample {
private static final String MODULUS = "FFFFFFFFFFFFFFFFC90F892B0DA4A2FC000756AC0710657B8E30038A8530FA773BBC9AB8514" +
"5EBFE70C5D8D31E0FFFA590C845BFEA3C14DBCF87DE8AECC1CBB82B5E1DBF1055ED"
// You can replace this with a larger prime number from RFC 3526
;
public static void main(String[] args) throws Exception {
BigInteger p = new BigInteger(MODULUS, 16);
// Generate or load DH parameters (from a trusted source)
DHParameterSpec dhParams = new DHParameterSpec(p, 2); // 2 is the generator (RFC 3526 uses 2)
// Alice and Bob agree on these parameters
Alice alice = new Alice(dhParams);
Bob bob = new Bob(dhParams);
BigInteger aliceSecret = alice.generatePrivateKey();
BigInteger bobSecret = bob.generatePrivateKey();
// Alice computes shared key (Bob's public key)
BigInteger sharedKeyAlice = alice.exchange(bob.getPublicKey());
// Bob computes shared key (Alice's public key)
BigInteger sharedKeyBob = bob.exchange(alice.getPublicKey());
// Both should get the same result
System.out.printf("Shared secret: %s%n", sharedKeyAlice.equals(sharedKeyBob) ? "Match" : "Mismatch");
}
static class Alice {
private final DHParameterSpec params;
public Alice(DHParameterSpec params) {
this.params = params;
}
public BigInteger generatePrivateKey() {
return new BigInteger(130, SecureRandom.getInstanceStrong()); // Just an example; use proper random generation in practice
}
public BigInteger getPublicKey() {
return params.getP().modPow(getPrivateKey(), params.getG());
}
BigInteger exchange(BigInteger remotePublicKey) {
return remotePublicKey.modPow(getPrivateKey(), params.getP());
}
}
static class Bob {
private final DHParameterSpec params;
public Bob(DHParameterSpec params) {
this.params = params;
}
// Similar to Alice, generates a random private key and computes the public key
// ... (omitted for brevity)
}
}
```
在这个例子中,Alice和Bob各自生成随机私钥,通过公钥交换计算出相同的共享密钥。请注意,实际应用中需要更安全的方式来生成随机数,并从可信源获取DH参数。
阅读全文
相关推荐
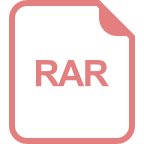
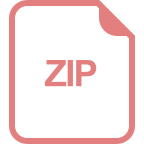
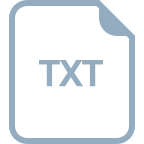
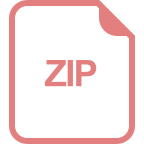
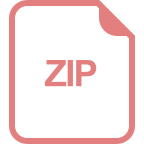
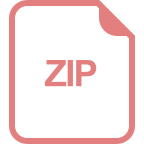
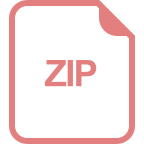
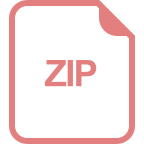
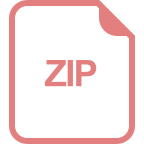
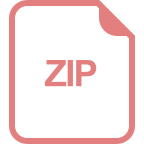
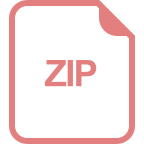
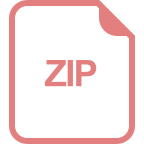
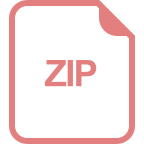
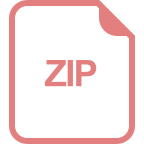
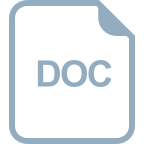
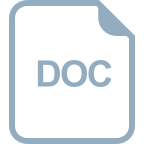