Main.java:1: error: class HelloJava is public, should be declared in a file named HelloJava.java public class HelloJava ^ 1 error
时间: 2024-09-11 22:08:07 浏览: 100
这个错误表明你在 `Main.java` 文件中声明了一个名为 `HelloJava` 的公共类,但Java的命名规则规定,如果一个类被声明为公共(public),那么它的源代码文件应该与类名完全一致,即应有一个单独的 `HelloJava.java` 文件来定义这个类。
Java中的类文件命名规则是小驼峰命名法(CamelCase),并且类文件名通常与类名保持一致,除非存在包名。在这种情况下,你应该将 `HelloJava` 类移动到另一个名为 `HelloJava.java` 的文件中,并确保 `Main.java` 文件中正确导入了这个类。
修复后的结构可能像这样:
- `src/com/example/Main.java`(如果有包)
```java
import com.example.HelloJava;
public class Main {
public static void main(String[] args) {
new HelloJava().sayHello();
}
}
```
- `src/com/example/HelloJava.java`
```java
public class HelloJava {
public void sayHello() {
System.out.println("你好,Java!");
}
}
```
相关问题
java declared_Java Class getDeclaredAnnotation()用法及代码示例
`getDeclaredAnnotation(Class<T> annotationClass)`方法可以用来获取指定注解类型的注解对象。它会从当前类的声明注解中查找,不会从父类中查找。
下面是一个简单的示例代码:
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@interface MyAnnotation {
String value();
}
@MyAnnotation("Hello World")
public class MyClass {
public static void main(String[] args) {
MyAnnotation annotation = MyClass.class.getDeclaredAnnotation(MyAnnotation.class);
System.out.println(annotation.value()); // 输出:Hello World
}
}
```
在这个示例中,我们定义了一个注解 `MyAnnotation`,并将其应用在 `MyClass` 类上。然后,在 `main` 方法中使用 `getDeclaredAnnotation` 方法获取 `MyAnnotation` 类型的注解对象,并输出注解中的 `value` 值。
需要注意的是,`getDeclaredAnnotation` 方法返回的是指定注解类型的注解对象,如果当前类中没有声明该注解,则返回 `null`。此外,在使用该方法时,需要通过泛型参数指定注解类型。
The main method header is writtens as
In Java, the `main` method serves as the entry point of a Java application when it's executed. It's where the execution begins, and it typically contains the first line of code that gets run. The syntax for a standard `main` method header in a Java class is as follows:
```java
public static void main(String[] args)
```
Here's what each part means:
1. **public**: This keyword makes the method accessible to other classes outside its package.
2. **static**: Since you can call a `main` method without creating an instance of the class, it needs to be declared as static.
3. **void**: The `main` method doesn't return any value, so it's declared as returning `void`.
4. **main**: This is the name of the method, which follows Java naming conventions (all lowercase with words separated by underscores).
5. **String[] args**: This parameter represents an array of strings called "command-line arguments." These are optional input values that can be passed to your program when executing from the command line.
To use this method, you typically write some Java code inside it that initializes your application, processes inputs, and performs necessary operations before exiting. For example:
```java
public static void main(String[] args) {
System.out.println("Hello, World!");
}
```
阅读全文
相关推荐
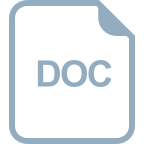
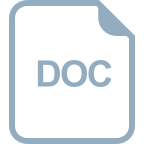
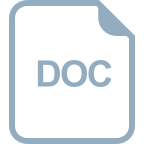
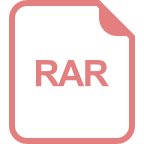
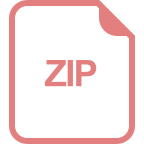
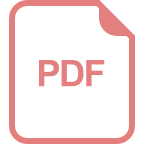
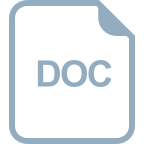
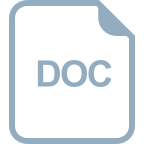
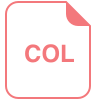
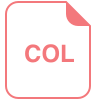
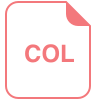
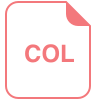
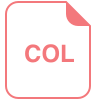
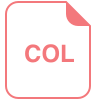
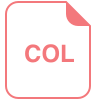
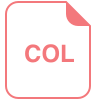
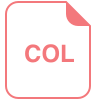