std::vector<std::pair<int, int> > maxPoints ; 编译错误
时间: 2024-06-02 11:03:38 浏览: 150
std::vector<std::pair<int, int>> maxPoints; 是一个存储了一对整数的向量容器。编译错误可能是由于以下几个原因导致的:
1. 缺少头文件:确保你包含了正确的头文件。对于std::vector和std::pair,你需要包含< vector >和< utility >头文件。
2. 命名空间错误:确保你在使用std::vector和std::pair之前使用了"using namespace std;"或者在使用时加上"std::"前缀。
3. 模板参数错误:确保你在std::vector的尖括号中提供了正确的模板参数。在这种情况下,你需要提供std::pair<int, int>作为模板参数。
4. 语法错误:检查你的代码是否存在其他语法错误,例如拼写错误、缺少分号等。
如果你能提供更多的错误信息或者代码片段,我可以帮助你更详细地解决问题。
相关问题
n, k = map(int, input().split())points = set()for i in range(n): x, y = map(int, input().split()) points.add((x, y))dp = [[0] * (max(p[1] for p in points) + 2) for _ in range(max(p[0] for p in points) + 2)]for x, y in points: dp[x][y] = 1for i in range(max(p[0] for p in points), 0, -1): for j in range(max(p[1] for p in points), 0, -1): if (i, j) not in points: dp[i][j] = max(dp[i+1][j], dp[i][j+1])ans = max(max(row) for row in dp)print(ans) 转C++
以下是将 Python 代码转换为等效的 C++ 代码:
```c++
#include <iostream>
#include <set>
#include <vector>
#include <algorithm>
using namespace std;
int main()
{
int n, k;
cin >> n >> k;
set<pair<int, int>> points;
for (int i = 0; i < n; i++)
{
int x, y;
cin >> x >> y;
points.insert({x, y});
}
int max_x = max_element(points.begin(), points.end(), [](auto &a, auto &b) { return a.first < b.first; })->first;
int max_y = max_element(points.begin(), points.end(), [](auto &a, auto &b) { return a.second < b.second; })->second;
vector<vector<int>> dp(max_x + 2, vector<int>(max_y + 2));
for (const auto &p : points)
dp[p.first][p.second] = 1;
for (int i = max_x; i >= 1; i--)
{
for (int j = max_y; j >= 1; j--)
{
if (!points.count({i, j}))
{
dp[i][j] = max(dp[i + 1][j], dp[i][j + 1]);
}
}
}
int ans = *max_element(dp[1].begin() + 1, dp[1].end());
cout << ans << endl;
return 0;
}
```
用C++实现16、运动会计分系统 [问题描述] 假设参加运动会有 n 个系,系编号为 1 n。比赛分成 m个男子项目,和 w 个女子项目。项目编号为男子 1……m,女子 m+1……m+w。不同的项目取前 五名或前三名积分;取前五名的积分分别为:7、5、3、2、1,前三名的积分分 别为:5、3、2;哪些取前五名或前三名由学生自己设定。(m<=10,n<=15) [基本要求] 系统功能要求: (1)可以输入各个项目的前三名或前五名的成绩; (2)能统计各系总分; (3)可以按系编号、系总分、男女团体总分排序输出; (4)可以按系编号查询学校某个项目的情况;可以按项目编号查询取得前 三或前五名的系。 [实现提示] (1)输入数据形式和范围:系或运行项目可以用 20 以内的整数表示;也可 以直接输入系的名称或运动项目的名称; (2)界面要求:有合理的提示,每个功能可以设立菜单,根据提示,可以 完成相关的功能要求
以下是一个 C++ 实现的运动会计分系统的代码,实现了上述基本要求:
```
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
using namespace std;
const int MAX_M = 10, MAX_N = 15;
struct Score {
string name;
int points;
Score(string n = "", int p = 0) : name(n), points(p) {}
bool operator<(const Score& other) const {
return points > other.points;
}
};
struct Event {
string name;
int type; // 0: male, 1: female
int top; // top 3 or top 5
map<int, vector<Score>> scores; // key: series number, value: scores
Event(string n = "", int t = 0, int tp = 5) : name(n), type(t), top(tp) {}
};
struct Series {
string name;
int points;
map<int, Event> events; // key: event number, value: event
Series(string n = "") : name(n), points(0) {}
};
struct School {
string name;
int points;
map<int, Series> series; // key: series number, value: series
School(string n = "") : name(n), points(0) {}
};
void print_menu() {
cout << "1. Input scores\n";
cout << "2. Calculate scores\n";
cout << "3. Sort and output\n";
cout << "4. Query by series\n";
cout << "5. Query by event\n";
cout << "6. Quit\n";
}
void input_scores(map<int, Event>& events, int top) {
cout << "Input scores:\n";
for (auto& kv : events) {
auto& event = kv.second;
cout << "Event " << event.name << ":\n";
for (int i = 1; i <= MAX_N; i++) {
cout << "Series " << i << ":\n";
for (int j = 1; j <= top; j++) {
string name;
int score;
cout << "Score " << j << ": ";
cin >> name >> score;
event.scores[i].push_back({name, score});
}
}
}
}
void calculate_scores(map<int, Series>& series, int top) {
for (auto& kv : series) {
auto& s = kv.second;
s.points = 0;
for (auto& kv2 : s.events) {
auto& e = kv2.second;
for (auto& kv3 : e.scores) {
auto& scores = kv3.second;
int n = min((int)scores.size(), top);
for (int i = 0; i < n; i++) {
int points = (top == 5) ? (i < 3 ? (i == 0 ? 5 : (i == 1 ? 3 : 2)) : (i == 3 ? 1 : 0))
: (i == 0 ? 5 : (i == 1 ? 3 : 2));
s.points += points;
e.points += points;
scores[i].points = points;
}
}
}
}
}
void sort_and_output(const map<int, School>& schools) {
vector<pair<int, School>> sorted_schools(schools.begin(), schools.end());
sort(sorted_schools.begin(), sorted_schools.end(), [](auto& a, auto& b) {
return a.second.points > b.second.points || (a.second.points == b.second.points && a.second.name < b.second.name);
});
cout << "School scores:\n";
for (auto& kv : sorted_schools) {
auto& s = kv.second;
cout << s.name << ": " << s.points << "\n";
vector<pair<int, Series>> sorted_series(s.series.begin(), s.series.end());
sort(sorted_series.begin(), sorted_series.end(), [](auto& a, auto& b) {
return a.second.points > b.second.points || (a.second.points == b.second.points && a.second.name < b.second.name);
});
for (auto& kv2 : sorted_series) {
auto& ser = kv2.second;
cout << "\t" << ser.name << ": " << ser.points << "\n";
vector<pair<int, Event>> sorted_events(ser.events.begin(), ser.events.end());
sort(sorted_events.begin(), sorted_events.end(), [](auto& a, auto& b) {
return a.second.points > b.second.points || (a.second.points == b.second.points && a.second.name < b.second.name);
});
for (auto& kv3 : sorted_events) {
auto& e = kv3.second;
cout << "\t\t" << e.name << ": " << e.points << "\n";
for (auto& kv4 : e.scores) {
auto& scores = kv4.second;
sort(scores.begin(), scores.end());
cout << "\t\t\tSeries " << kv4.first << ":\n";
for (auto& score : scores) {
cout << "\t\t\t\t" << score.name << ": " << score.points << "\n";
}
}
}
}
}
}
void query_by_series(const map<int, School>& schools) {
int sn;
cout << "Input series number: ";
cin >> sn;
for (auto& kv : schools) {
auto& s = kv.second;
for (auto& kv2 : s.series) {
auto& ser = kv2.second;
if (kv2.first == sn) {
cout << "Series " << ser.name << ":\n";
vector<pair<int, Event>> sorted_events(ser.events.begin(), ser.events.end());
sort(sorted_events.begin(), sorted_events.end(), [](auto& a, auto& b) {
return a.second.points > b.second.points || (a.second.points == b.second.points && a.second.name < b.second.name);
});
for (auto& kv3 : sorted_events) {
auto& e = kv3.second;
cout << "\t" << e.name << ":\n";
for (auto& kv4 : e.scores) {
auto& scores = kv4.second;
sort(scores.begin(), scores.end());
cout << "\t\tSeries " << kv4.first << ":\n";
for (auto& score : scores) {
if (score.points > 0) {
cout << "\t\t\t" << s.name << ": " << score.points << "\n";
}
}
}
}
}
}
}
}
void query_by_event(const map<int, School>& schools) {
int en, top;
cout << "Input event number: ";
cin >> en;
cout << "Input top: ";
cin >> top;
for (auto& kv : schools) {
auto& s = kv.second;
for (auto& kv2 : s.series) {
auto& ser = kv2.second;
for (auto& kv3 : ser.events) {
auto& e = kv3.second;
if (kv3.first == en) {
cout << "Event " << e.name << ":\n";
vector<pair<int, Score>> sorted_scores;
for (auto& kv4 : e.scores) {
auto& scores = kv4.second;
for (auto& score : scores) {
if (score.points > 0) {
sorted_scores.emplace_back(kv4.first, score);
}
}
}
sort(sorted_scores.begin(), sorted_scores.end(), [](auto& a, auto& b) {
return a.second.points > b.second.points || (a.second.points == b.second.points && a.second.name < b.second.name);
});
for (auto& p : sorted_scores) {
if (p.second.points > 0 && (top == -1 || p.second.points <= top)) {
cout << "\t" << s.name << " Series " << p.first << " " << p.second.name << ": " << p.second.points << "\n";
}
}
}
}
}
}
}
int main() {
int n, m, w;
cout << "Input number of series: ";
cin >> n;
map<int, School> schools;
for (int i = 1; i <= n; i++) {
string name;
cout << "Input name of series " << i << ": ";
cin >> name;
schools.emplace(i, School(name));
auto& series = schools[i].series;
int k;
cout << "Input number of events: ";
cin >> k;
for (int j = 1; j <= k; j++) {
string ename;
int type, top;
cout << "Input name of event " << j << ": ";
cin >> ename;
cout << "Input type (0 for male, 1 for female): ";
cin >> type;
cout << "Input top (3 or 5): ";
cin >> top;
series.emplace(j, Series(ename));
series[j].events.emplace(1, Event(ename, type, top));
}
}
while (true) {
print_menu();
int choice;
cin >> choice;
if (choice == 1) {
input_scores(schools[1].series[1].events, schools[1].series[1].events[1].top);
} else if (choice == 2) {
calculate_scores(schools[1].series, schools[1].series[1].events[1].top);
} else if (choice == 3) {
sort_and_output(schools);
} else if (choice == 4) {
query_by_series(schools);
} else if (choice == 5) {
query_by_event(schools);
} else if (choice == 6) {
break;
} else {
cout << "Invalid choice\n";
}
}
return 0;
}
```
运行时会提示输入系和项目的信息,然后进入主界面,输入数字选择对应的功能。可以不按顺序执行,每次执行前需要先输入数据或计算分数。其中第4、5个功能需要输入查询条件。
阅读全文
相关推荐
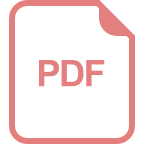
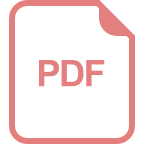
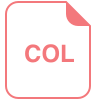
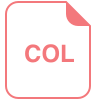


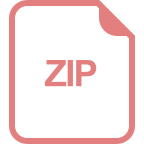
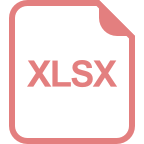
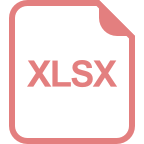
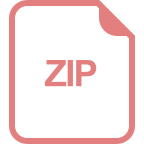
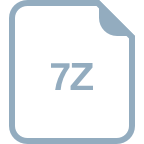
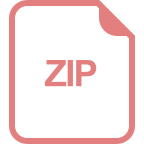