C++ 二维指针 _aligned_malloc 内存申请及释放
时间: 2024-10-25 09:03:05 浏览: 23
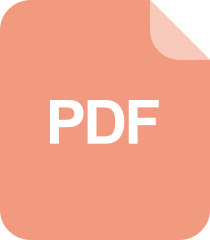
深入解析C++编程中__alignof 与__uuidof运算符的使用
`_aligned_malloc` 是C++标准库中的一个非成员函数,它允许开发者在特定对齐边界上动态分配内存。这个函数通常用于需要特定数据结构对齐需求的情况,例如处理浮点数、矩阵运算等,浮点数通常需要16字节的对齐。
`_aligned_malloc(size_t size, size_t alignment)` 的作用是:
1. `size` 参数表示要分配的字节数量。
2. `alignment` 参数指定所需的内存对齐,比如16、32或64字节。
使用示例:
```cpp
#include <cstdlib>
#include <cstdint>
// 指定8字节对齐
void* alignedMalloc(size_t size) {
return _aligned_malloc(size, sizeof(void*) * 8); // 通常是系统最大对齐值
}
// 分配一个二维数组,假设每个元素是一个double
void* allocateMatrix(int rows, int cols) {
return alignedMalloc(rows * cols * sizeof(double));
}
// 使用分配的内存
void workWithMatrix(double** matrix, int rows, int cols) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
matrix[i][j] = 0.0;
}
}
}
// 当不再需要内存时释放
void alignedFree(void* ptr) {
if (ptr != nullptr) {
_aligned_free(ptr);
}
}
int main() {
double** mat = static_cast<double*>(alignedMalloc(10 * 10)); // 分配一个10x10矩阵
// ... 使用矩阵
alignedFree(mat); // 释放内存
return 0;
}
```
阅读全文
相关推荐
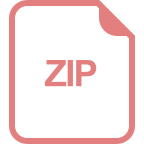




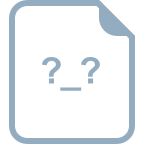
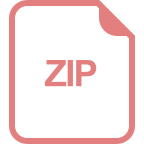









