python如何进入shell执行adb命令
时间: 2024-09-10 08:13:32 浏览: 59
在Python中进入shell执行ADB(Android Debug Bridge)命令,你可以使用`os`模块中的`system`函数或者`subprocess`模块中的`Popen`、`call`、`check_output`等函数。以下是两种常用的方法:
1. 使用`os.system`方法:
```python
import os
os.system("adb devices") # 查询已连接设备
```
2. 使用`subprocess`模块:
```python
import subprocess
# 使用Popen方法执行adb命令,获取设备列表
process = subprocess.Popen("adb devices", stdout=subprocess.PIPE, shell=True)
output, error = process.communicate()
print(output.decode()) # 输出执行结果
# 使用check_output方法执行adb命令,直接获取结果字符串
result = subprocess.check_output("adb devices", shell=True)
print(result.decode()) # 输出执行结果
```
注意,使用`subprocess`模块比`os.system`更加灵活且强大,可以更方便地处理命令执行的输入输出和错误处理。
相关问题
python 如何进入adb shell执行命令
在 Python 中可以使用 `subprocess` 模块来执行 adb 命令,并进入 adb shell 执行命令。示例如下:
```python
import subprocess
# 执行 adb shell 命令
p = subprocess.Popen(['adb', 'shell'], stdin=subprocess.PIPE, stdout=subprocess.PIPE)
# 执行命令
out, err = p.communicate('ls /sdcard\n'.encode())
print(out.decode())
```
其中,`Popen` 函数用于创建一个新的进程,并返回一个 `Popen` 对象。`stdin=subprocess.PIPE` 表示将子进程的标准输入重定向到管道,`stdout=subprocess.PIPE` 表示将子进程的标准输出重定向到管道。然后可以使用 `communicate` 方法向子进程的标准输入发送命令,并获取子进程的标准输出和标准错误输出。示例中的命令是 `ls /sdcard\n`,表示在 adb shell 中执行 `ls /sdcard` 命令。注意,命令需要以换行符结尾。
python如何并行执行adb命令
在Python中,并行执行ADB(Android Debug Bridge)命令可以利用`concurrent.futures`模块,如`ThreadPoolExecutor`或`ProcessPoolExecutor`。这里是一个简单的例子,展示如何使用`subprocess`库结合这两个池来并发地执行ADB命令:
```python
import subprocess
from concurrent.futures import ThreadPoolExecutor
def run_adb_command(device, command):
# 使用Popen创建一个进程执行ADB命令
adb_cmd = ['adb', '-s', device, command]
try:
output = subprocess.check_output(adb_cmd, stderr=subprocess.STDOUT)
return output.decode('utf-8')
except subprocess.CalledProcessError as e:
print(f"Command failed with error: {e}")
# 设定设备列表和命令
devices = ['device1', 'device2'] # 根据实际需要替换为你的设备ID
command = 'shell ls' # ADB命令示例
with ThreadPoolExecutor(max_workers=len(devices)) as executor:
future_to_device = {executor.submit(run_adb_command, device, command): device for device in devices}
for future in concurrent.futures.as_completed(future_to_device):
device = future_to_device[future]
try:
result = future.result()
print(f"Device {device}: {result}")
except Exception as exc:
print(f"{device} raised an exception: {exc}")
阅读全文
相关推荐
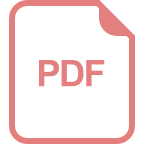
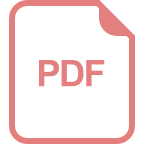
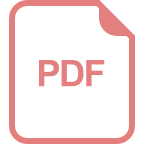













