如果我想从 std::string str(["A", "2", "A", "2"]) 中获得新的字符串"A2A2",应该用c++的正则匹配怎么做
时间: 2024-09-11 16:15:34 浏览: 45
在C++中,要从一个包含字符和数字的字符串中匹配并提取特定模式的字符序列,可以使用`<regex>`库中的正则表达式功能。如果你的目标是从字符串`"A2A2"`中提取所有的"A"字符和"2"数字,并将它们合并成一个新的字符串"A2A2",你可以使用正则表达式来匹配每个"A"后跟着一个"2"的模式,并将匹配到的字符串拼接起来。
下面是一个使用C++标准库中的`std::regex`进行匹配并生成结果字符串的示例代码:
```cpp
#include <iostream>
#include <string>
#include <regex>
int main() {
std::string str("A2A2");
std::regex pattern(R"((A2))"); // 正则表达式匹配"A2"模式
std::string result;
// 使用std::regex_iterator迭代器进行匹配
auto begin = std::sregex_iterator(str.begin(), str.end(), pattern);
auto end = std::sregex_iterator();
// 遍历所有匹配的结果
for (std::sregex_iterator i = begin; i != end; ++i) {
std::smatch match = *i;
result += match.str(); // 将匹配到的字符串添加到结果字符串中
}
std::cout << "Resulting string: " << result << std::endl; // 输出结果字符串
return 0;
}
```
在这段代码中,我们首先定义了要处理的字符串`str`和正则表达式`pattern`。我们使用`std::regex`构造函数创建了一个正则对象,并用`std::sregex_iterator`来遍历所有匹配的结果。然后将每个匹配到的字符串拼接起来,形成最终的结果。
运行上述代码,将会输出:
```
Resulting string: A2A2
```
阅读全文
相关推荐
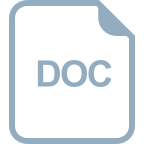
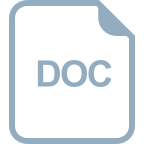
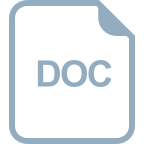

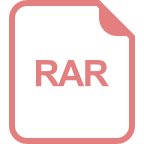
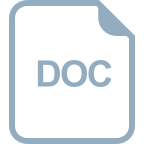
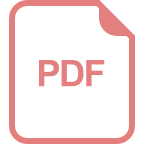
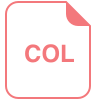
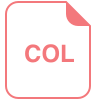
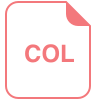









