c++实现json封装
时间: 2024-10-24 20:13:20 浏览: 14
在C++中,JSON(JavaScript Object Notation)是一种常用的数据交换格式,为了方便处理JSON数据,开发者通常会利用现成的库如RapidJSON、nlohmann/json等。这里简单介绍nlohmann/json库的使用:
nlohmann/json是一个轻量级且易于使用的C++ JSON库。首先,你需要从GitHub上下载并包含它的源码头文件。以下是基本的步骤:
1. **添加依赖**:如果你的项目支持CMake,可以在CMakeLists.txt中添加以下行来找到并链接nlohmann/json库:
```cmake
find_package(nlohmann_json REQUIRED)
target_link_libraries(your_project nlohmann_json::nlohmann_json)
```
2. **包含头文件**:
```cpp
#include <nlohmann/json.hpp>
using json = nlohmann::json;
```
3. **创建和操作JSON对象**:
- 创建JSON对象:
```cpp
json j; // 空对象
json j_obj = {{"key", "value"}, {"another_key", 42}}; // 定义对象
```
- 解析JSON字符串:
```cpp
std::string jsonString = R"({"name": "John", "age": 30})";
json jParsed = json::parse(jsonString);
```
- 序列化到字符串:
```cpp
std::string jsonString = jParsed.dump(); // 将JSON对象转换为字符串
```
4. **读取和写入文件**:
```cpp
std::ofstream file("data.json");
file << jParsed.dump(4); // 4表示缩进级别
file.close();
```
5. **访问元素**:
```cpp
std::string name = jParsed["name"].get<std::string>(); // 获取键值对
int age = jParsed["age"];
```
阅读全文
相关推荐
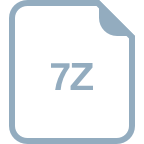















