用JavaWeb做book和author的列表功能,其中book和author都包含id和name的代码
时间: 2024-10-18 11:16:45 浏览: 11
在Java Web中,实现book和author的列表功能通常涉及到JSP、Servlet和数据库操作。这里是一个简单的示例,假设我们使用了Spring MVC框架和MySQL数据库:
首先,创建Book实体类(Book.java):
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters
}
```
然后,Author实体类(Author.java)类似:
```java
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// getters and setters
}
```
创建对应的Repository接口(如BookRepository.java):
```java
import org.springframework.data.jpa.repository.JpaRepository;
public interface BookRepository extends JpaRepository<Book, Long> {
}
```
同样,对于AuthorRepository。
接下来,在Service层(BookService.java)处理业务逻辑:
```java
import org.springframework.beans.factory.annotation.Autowired;
import java.util.List;
@Service
public class BookService {
@Autowired
private BookRepository bookRepository;
public List<Book> getAllBooks() {
return bookRepository.findAll();
}
public List<Author> getAllAuthors() {
return authorRepository.findAll();
}
}
```
最后,在控制器层(Controller)处理HTTP请求:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/api")
public class BookController {
@Autowired
private BookService bookService;
@GetMapping("/books")
public List<Book> getAllBooks() {
return bookService.getAllBooks();
}
@GetMapping("/authors")
public List<Author> getAllAuthors() {
return bookService.getAllAuthors();
}
}
```
在JSP页面上显示列表:
```jsp
<table>
<tr th:each="book : ${books}">
<td th:text="${book.name}">Book Name</td>
<!-- 更多列... -->
</tr>
</table>
<!-- authors表同理 -->
```
这只是一个基本的示例,实际应用中可能还需要分页、排序、搜索等功能,并且数据可能存储在更复杂的数据库结构中。
阅读全文
相关推荐
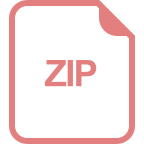
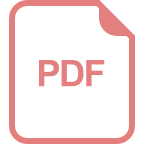
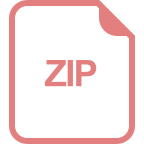


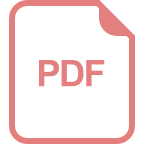












