cpp 计算2的n次方的后三位
时间: 2024-10-06 20:04:15 浏览: 34
在C++中,计算2的n次方的后三位并不复杂,但随着n的增长,我们需要考虑性能优化,因为直接进行乘法运算可能会导致数值溢出。一种常见的做法是利用位操作和取模来限制结果的范围。以下是基本的思路:
```cpp
#include <iostream>
#include <bitset>
std::string calculate_last_three_bits(int n) {
std::string result = "000"; // 初始化为三个零
unsigned long long int power_of_two = 1; // 开始计算,初始值为1
while (power_of_two <= static_cast<unsigned long long int>(pow(10, 3))) { // 只需考虑后三位
power_of_two *= 2;
if ((n % 4 == 0) || (n % 4 == 1)) { // 如果 n 能被4整除或余1,保留当前位
result[0] = char(power_of_two & 15); // 最后一位
result[1] = char((power_of_two >> 1) & 15); // 第二位
result[2] = char((power_of_two >> 2) & 15); // 第三位
}
n >>= 2; // 把 n 向右移动两位,处理下一个四位一组
}
return result;
}
int main() {
int n;
std::cout << "Enter a value for n: ";
std::cin >> n;
std::cout << "The last three bits of 2 to the power of " << n << " are: " << calculate_last_three_bits(n) << std::endl;
return 0;
}
```
在这个代码里,我们首先创建了一个空字符串`result`来保存后三位,然后用`power_of_two`变量存储2的递增幂次。通过位与操作(`&`)和位移操作(`>>`),我们可以获取并保留每个位置的数字。对于每次循环,我们将n除以4并将余数检查是否对应于特定的末尾位情况。
阅读全文
相关推荐
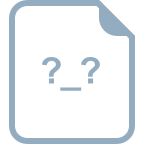
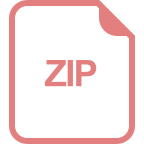















