c++ 10的n次方
时间: 2024-09-17 08:08:43 浏览: 33
在C++10标准中,并没有直接提供内置的运算符来计算幂(即n次方)。不过,你可以通过`std::pow`函数或者自定义函数模板来实现这个功能。`std::pow`是C++ `<cmath>`头文件里的成员函数,它接受两个double类型的参数,第一个是底数,第二个是指数。
```cpp
#include <cmath> // 引入math库
// 使用std::pow计算n次方
double power(double base, int exponent) {
return std::pow(base, static_cast<double>(exponent));
}
```
如果你需要支持更大的整数,可以创建一个自定义模板函数,例如:
```cpp
template <typename T>
T pow(T base, T exponent) {
T result = 1;
while (exponent > 0) {
if (exponent & 1) // 如果指数是奇数
result *= base;
base *= base; // 将基数平方
exponent >>= 1; // 右移一位相当于除以2
}
return result;
}
```
在这个模板函数中,我们利用了位操作来简化计算过程。
相关问题
C++中计算10的n次方
在C++中,你可以使用循环或递归的方式来计算10的n次方。这里我们提供两种简单的方法:
**使用乘法循环**
```cpp
#include<iostream>
using namespace std;
int power(int base, int exponent) {
if (exponent == 0) // 防止除以0错误
return 1;
else if (exponent > 0)
return base * power(base, exponent - 1);
else // exponent为负的情况
return 1 / base; // 这里实际上不会执行,因为我们会先处理exponent为正的情况
}
int main() {
int n;
cout << "Enter an integer power: ";
cin >> n;
long long result = power(10, n);
cout << "10 to the power of " << n << " is: " << result << endl;
return 0;
}
```
**使用模板库中的pow函数(C++11及以上版本)**
```cpp
#include<iostream>
#include<cmath> // 引入math头文件,包含pow函数
int main() {
int n;
cout << "Enter an integer power: ";
cin >> n;
double result = pow(10.0, n); // 使用double类型防止精度丢失
cout << "10 to the power of " << n << " is: " << result << endl;
return 0;
}
```
这两种方法都会避免直接对大整数进行幂运算,提高效率。
C++ 2的n次方 库函数
在C++中,可以使用库函数pow来计算一个数的幂。在你提供的代码中,可以看到使用了pow函数来计算n的m次方。具体代码如下:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int n;
cin >> n;
cout << pow(2, n) << endl;
return 0;
}
```
这段代码中,我们使用了cmath头文件中的pow函数来计算2的n次方。输入一个整数n,然后调用pow函数,将2和n作为参数传入,得到2的n次方的结果,并输出。
#### 引用[.reference_title]
- *1* [C++中开n次方的方式](https://blog.csdn.net/starlet_kiss/article/details/105916700)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [求x的n次方(x^n) 库函数power](https://blog.csdn.net/weixin_64954259/article/details/123600179)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
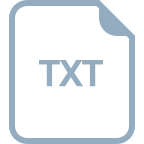
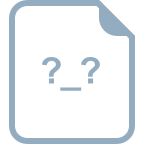












