Java 编写一个程序,完成从某账户取款和存款的操作。 1)输人存取款金额时,如果非数值型,捕获异常并进行处理(系统异常)。 2)操作账户类时,如果取款数大于余额则作为异常处理(自定义异常)。
时间: 2024-10-22 11:25:42 浏览: 28
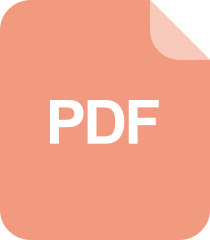
JAVA实现账户取款和存款操作
Java是一种广泛使用的高级编程语言,它由Sun Microsystems(现已被Oracle收购)开发,并后来成为Oracle的一部分。Java以其"一次编写,到处运行"的理念而知名,因为它具备平台无关性,即Java程序可以在任何支持Java的平台上运行,无需对底层操作系统做调整。
在这个任务中,我们可以创建一个简单的银行账户类BankAccount,包含私有变量如balance表示余额,以及两个方法deposit(存款)和withdraw(取款)。以下是相关的Java代码示例:
```java
import java.util.Scanner;
import java.text.DecimalFormat;
class InsufficientBalanceException extends Exception {
public InsufficientBalanceException(String message) {
super(message);
}
}
public class BankAccount {
private double balance;
public BankAccount(double initialBalance) {
this.balance = initialBalance;
}
// 存款方法
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
System.out.println("Deposit successful. New balance: " + new DecimalFormat("#.##").format(balance));
} else {
throw new IllegalArgumentException("Deposit amount must be positive.");
}
}
// 取款方法
public void withdraw(double amount) throws InsufficientBalanceException {
if (amount > 0 && balance >= amount) {
balance -= amount;
System.out.println("Withdrawal successful. New balance: " + new DecimalFormat("#.##").format(balance));
} else {
if (amount <= 0) {
throw new IllegalArgumentException("Withdrawal amount must be positive.");
} else {
throw new InsufficientBalanceException("Insufficient balance to withdraw requested amount.");
}
}
}
}
public class Main {
public static void main(String[] args) {
BankAccount account = new BankAccount(1000); // 初始化账户
Scanner scanner = new Scanner(System.in);
System.out.print("Enter deposit or withdrawal amount: ");
String input = scanner.nextLine();
try {
double amount = Double.parseDouble(input);
if ("deposit".equals(input.toLowerCase())) {
account.deposit(amount);
} else if ("withdraw".equals(input.toLowerCase())) {
account.withdraw(amount);
} else {
System.out.println("Invalid operation. Please enter 'deposit' or 'withdraw'.");
}
} catch (NumberFormatException e) {
System.out.println("Error: Invalid input. Please enter a numeric value.");
} catch (InsufficientBalanceException e) {
System.out.println(e.getMessage());
}
}
}
```
这个程序首先创建了一个BankAccount实例,然后通过Scanner获取用户输入,尝试转换为数字进行操作。如果输入不是数字或者取款金额超过余额,会抛出相应的异常并进行处理。
阅读全文
相关推荐
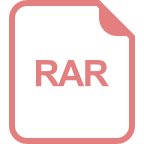
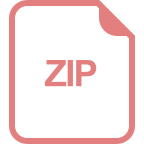















