使用c++编写一个类date,里面的内容有年月日,有重载运算符+,-,++,--
时间: 2024-10-25 07:18:25 浏览: 52
在C++中,我们可以创建一个名为Date的类来表示日期,包含年、月、日成员变量,并重载加减运算符(+,-,++,--)。下面是一个简单的示例:
```cpp
#include <iostream>
class Date {
private:
int year;
int month;
int day;
public:
// 构造函数
Date(int y = 0, int m = 0, int d = 0) : year(y), month(m), day(d) {}
// 访问器
int getYear() const { return year; }
int getMonth() const { return month; }
int getDay() const { return day; }
// 重载运算符+
Date operator+(const Date& other) {
int new_year = year + other.year;
int new_month = (month + other.month + other.day > 30) ? (month + other.month + other.day - 30) : (month + other.month + other.day);
int new_day = (new_month == 13 || (new_month == 2 && !is_leap_year(year))) ? 1 : (day + other.day);
if (is_leap_year(year) && month == 2 && new_day > 29) new_day -= 1;
return Date(new_year, new_month % 12, new_day);
}
// 其他运算符可以类似重载
Date operator-(const Date& other) { ... } // 减法
Date operator++() { ... } // 自增
Date operator--() { ... } // 自减
// 辅助函数判断是否为闰年
bool is_leap_year(int year) const {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
};
// 示例
int main() {
Date date1(2023, 2, 28);
Date date2(2023, 3, 1);
Date sum = date1 + date2; // 调用自定义的+运算符
std::cout << "Sum of dates: " << sum.getYear() << "/" << sum.getMonth() << "/" << sum.getDay() << "\n";
return 0;
}
```
阅读全文
相关推荐
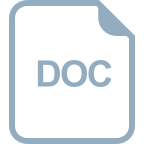
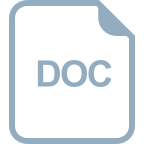
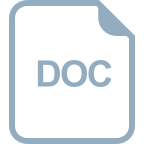
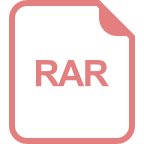
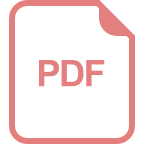
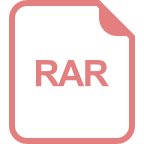
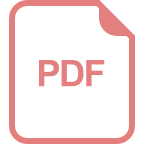
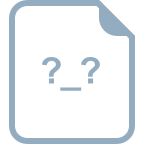
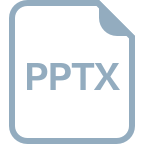
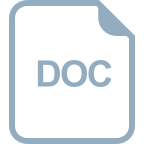
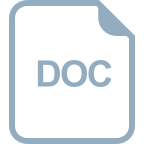
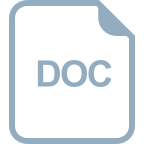
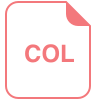




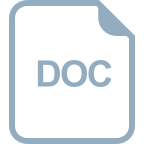
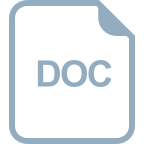