算术表达式求值演示数据结构代码
时间: 2023-12-22 10:30:12 浏览: 98
以下是一个算术表达式求值的演示代码,使用数据结构中的栈来实现:
```python
class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return len(self.items) == 0
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
def peek(self):
if not self.is_empty():
return self.items[-1]
def size(self):
return len(self.items)
def evaluate_expression(expression):
operators = {'+': 1, '-': 1, '*': 2, '/': 2}
operand_stack = Stack()
operator_stack = Stack()
for char in expression:
if char.isdigit():
operand_stack.push(int(char))
elif char in operators:
while (not operator_stack.is_empty() and
operators[char] <= operators[operator_stack.peek()]):
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
operator = operator_stack.pop()
result = perform_operation(operand1, operand2, operator)
operand_stack.push(result)
operator_stack.push(char)
while not operator_stack.is_empty():
operand2 = operand_stack.pop()
operand1 = operand_stack.pop()
operator = operator_stack.pop()
result = perform_operation(operand1, operand2, operator)
operand_stack.push(result)
return operand_stack.pop()
def perform_operation(operand1, operand2, operator):
if operator == '+':
return operand1 + operand2
elif operator == '-':
return operand1 - operand2
elif operator == '*':
return operand1 * operand2
elif operator == '/':
return operand1 / operand2
expression = "3+4*2-6/3"
result = evaluate_expression(expression)
print("Result:", result) # 输出:7
```
阅读全文
相关推荐



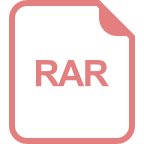

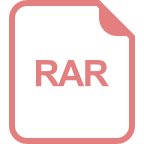

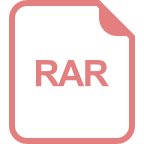

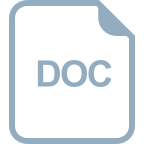


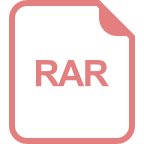
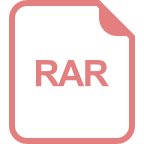



