if getattr(instance, '_prefetched_objects_cache', None): instance._prefetched_objects_cache = {}
时间: 2024-04-12 16:32:42 浏览: 14
这段代码用于清空实例对象的 `_prefetched_objects_cache` 属性。
在 Django ORM 中,使用 `select_related` 和 `prefetch_related` 方法可以优化查询性能,减少数据库查询次数。这些方法会将相关对象预先查询并缓存到 `_prefetched_objects_cache` 属性中,以便在后续访问时可以直接从缓存中获取,而不再进行数据库查询。
在给定的代码中,通过使用 `getattr()` 函数,检查实例对象是否有 `_prefetched_objects_cache` 属性。如果存在该属性并且不为 `None`,则将其赋值为空字典 `{}`,即清空缓存。
这段代码的作用是在更新操作完成后,清除实例对象的缓存,以便在后续访问时重新查询相关对象。这可能是为了避免在后续操作中使用过期或无效的缓存数据,并确保获取最新的数据。
相关问题
def update(self, request, *args, **kwargs): partial = kwargs.pop('partial', False) instance = self.get_object() serializer = self.get_serializer(instance, data=request.data, partial=partial) serializer.is_valid(raise_exception=True) self.perform_update(serializer) if getattr(instance, '_prefetched_objects_cache', None): instance._prefetched_objects_cache = {} return APIResponse(msg="已更新", result=serializer.data)
这段代码是一个视图方法,用于处理 HTTP PUT 或 PATCH 请求,即更新资源的请求。
在方法中,首先从 `kwargs` 字典中弹出 `partial` 键对应的值,默认为 `False`。然后,通过调用 `self.get_object()` 方法获取要更新的实例对象。接下来,使用请求数据和实例对象创建一个序列化器(serializer),并设置 `partial` 参数为之前弹出的值。然后,通过调用 `serializer.is_valid(raise_exception=True)` 方法对序列化器进行验证,如果验证失败则会抛出异常。
之后,调用 `self.perform_update(serializer)` 方法执行实际的更新操作。如果实例对象有 `_prefetched_objects_cache` 属性,则将其置为空字典。
最后,返回一个包含响应消息和序列化器数据的 API 响应对象,响应消息为 "已更新",响应结果为序列化器的数据。
总的来说,这段代码实现了更新操作的逻辑,包括数据验证、执行更新操作和返回响应。具体实现和使用需要结合代码上下文进行进一步理解。
self.__getattr__
self.__getattr__ is a special method in Python that is used to define what happens when an attribute that does not exist is accessed on an object. It is called automatically by Python when an attribute lookup fails, and it can be used to dynamically generate attributes or to redirect attribute access to other objects.
For example, let's say we have a class called Person that has a dictionary attribute called info. We can use __getattr__ to allow accessing the keys of the info dictionary directly on the Person object:
```
class Person:
def __init__(self, name):
self.name = name
self.info = {'age': 30, 'city': 'New York'}
def __getattr__(self, name):
if name in self.info:
return self.info[name]
else:
raise AttributeError(f"'{self.__class__.__name__}' object has no attribute '{name}'")
```
Now we can create a Person object and access the age and city attributes directly:
```
p = Person('John')
print(p.age) # Output: 30
print(p.city) # Output: New York
```
If we try to access an attribute that doesn't exist in the info dictionary, we'll get an AttributeError:
```
print(p.gender) # Output: AttributeError: 'Person' object has no attribute 'gender'
```
相关推荐
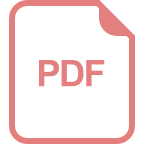
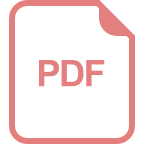
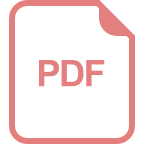












