在C语言中,如何利用POSIX线程库(pthreads)创建一个线程,并确保线程间的数据同步与安全性?请提供示例代码。
时间: 2024-12-01 11:13:50 浏览: 21
在C语言中进行多线程编程时,使用POSIX线程库(pthreads)是非常常见的方式。pthreads库为开发者提供了丰富的接口来创建和管理线程,同时包含各种同步机制,以确保线程间的数据同步与安全。
参考资源链接:[C语言多线程编程实战指南](https://wenku.csdn.net/doc/2p1h9ff2j0?spm=1055.2569.3001.10343)
首先,要创建一个线程,我们需要包含头文件`pthread.h`,然后使用`pthread_create()`函数。这个函数的原型如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
这里的参数分别代表线程指针、线程属性、线程启动函数和传递给启动函数的参数。
例如,下面的代码展示了如何创建一个线程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void* thread_function(void* arg) {
printf(
参考资源链接:[C语言多线程编程实战指南](https://wenku.csdn.net/doc/2p1h9ff2j0?spm=1055.2569.3001.10343)
相关问题
如何在C语言中利用POSIX线程库(pthreads)创建一个线程,并确保线程间的数据同步与安全性?请提供示例代码。
在C语言中使用POSIX线程库(pthreads)创建线程并通过同步机制保证数据安全性是多线程编程中的核心技能。为了帮助你更深入地理解这一过程,并提供一个操作性的示例,推荐参阅《C语言多线程编程实战指南》。这份资料详细介绍了C语言环境下多线程编程的各个环节,包含丰富的代码示例和实用的技术细节,直接关联到你当前的问题。
参考资源链接:[C语言多线程编程实战指南](https://wenku.csdn.net/doc/2p1h9ff2j0?spm=1055.2569.3001.10343)
线程的创建可以通过pthread_create函数实现。以下是创建线程并执行特定任务的基本示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
// 定义线程要执行的函数类型
void *thread_function(void *arg) {
// 线程执行的代码
printf(
参考资源链接:[C语言多线程编程实战指南](https://wenku.csdn.net/doc/2p1h9ff2j0?spm=1055.2569.3001.10343)
C语言如何利用pthreads库创建线程?
在C语言中,使用pthreads(POSIX Threads)库创建线程通常需要以下几个步骤:
1. **包含头文件**:首先在源文件中包含`pthread.h`头文件,它包含了所有与线程相关的函数声明。
```c
#include <pthread.h>
```
2. **定义线程函数**:这是每个线程将执行的任务,通常接收一个指向数据结构的指针作为参数,并通过`void *thread_func(void *arg)`这样的形式定义。
3. **创建线程**:使用`pthread_create()`函数创建新线程。这个函数需要四个参数:
- `pthread_t *thread`:返回线程标识符的指针。
- `pthread_attr_t *attr`:线程属性设置,可以指定优先级、栈大小等,这里一般设为NULL表示默认属性。
- `void *(*start_routine)(void *)`:线程函数的地址。
- `void *arg`:传给线程函数的参数。
示例:
```c
pthread_t thread_id;
pthread_attr_t attr;
pthread_create(&thread_id, &attr, thread_func, (void*)data);
```
4. **同步线程**:如果需要控制线程之间的交互,可以使用互斥锁(mutex)、条件变量(condition variable)等同步工具,如`pthread_mutex_lock()`和`pthread_cond_wait()`。
5. **销毁线程**:完成任务后,需要调用`pthread_join()`等待线程结束,然后清理资源。
```c
pthread_join(thread_id, NULL); // 等待线程结束
pthread_exit(NULL); // 如果线程自身结束,释放资源
```
6. **处理错误**:务必检查`pthread_create()`和`pthread_join()`等函数的返回值,因为它们可能会返回错误码。
```c
if (pthread_create(&thread_id, &attr, thread_func, (void*)data) != 0) {
// 错误处理
}
```
阅读全文
相关推荐
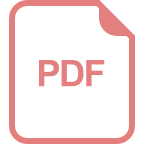
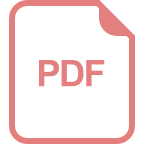
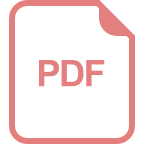
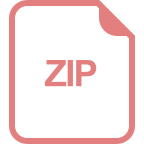
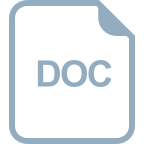
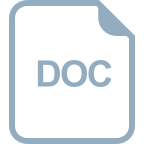
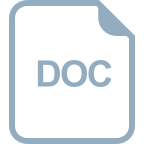
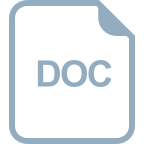
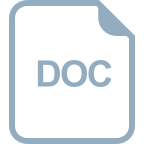
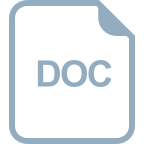
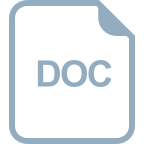
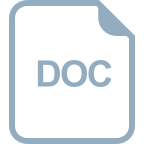
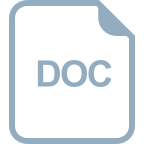
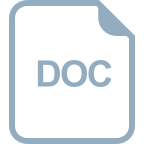
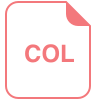

