sift特征提取python代码
时间: 2024-09-22 18:05:13 浏览: 32
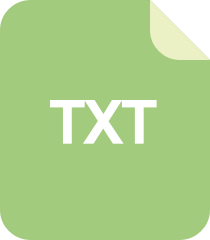
Sift特征提取——python代码实现
SIFT(Scale-Invariant Feature Transform,尺度不变特征变换)是一种常用的图像特征检测和描述算法。在Python中,我们可以使用开源库如`opencv-python`来实现SIFT。以下是简单的SIFT特征提取步骤的代码示例:
```python
import cv2
from skimage.feature import match_descriptors
# 加载图片
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 初始化SIFT
sift = cv2.xfeatures2d.SIFT_create()
# 找到关键点及其描述符
keypoints, descriptors = sift.detectAndCompute(img, None)
# 可选地,对关键点进行非极大值抑制,减少重复
keypoints = cv2.KeyPoint_convert(keypoints)
good_keypoints = cv2.cornerSubPix(img, keypoints, (5, 5), (-1, -1), criteria)
# 显示原始图像和关键点位置
cv2.drawKeypoints(img, good_keypoints, img, color=(0, 255, 0))
# 保存关键点文件
with open('keypoints.pkl', 'wb') as f:
pickle.dump(good_keypoints, f)
# 如果有另一张待匹配的图片
match_img = cv2.imread('other_image.jpg', cv2.IMREAD_GRAYSCALE)
_, other_descriptors = sift.detectAndCompute(match_img, None)
# 匹配描述符
matches = match_descriptors(descriptors, other_descriptors, crossCheck=True)
# 显示匹配结果
draw_params = dict(matchColor=(0, 255, 0),
singlePointColor=None,
matchesMask=matches,
flags=2)
img_matches = cv2.drawMatchesKnn(img, good_keypoints, match_img, None, matches, None, **draw_params)
cv2.imshow('Matched Images', img_matches)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
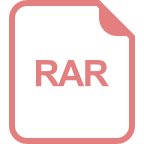


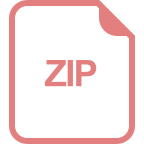
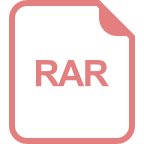
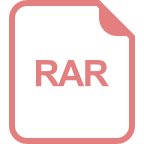




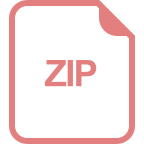
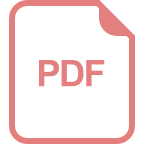
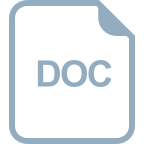
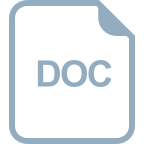
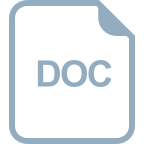

