一元多项式的相加用c语言实现
时间: 2024-11-23 12:30:44 浏览: 0
在C语言中,一元多项式相加通常涉及到处理系数数组和指数。这里是一个简单的例子,展示了如何将两个一元多项式(例如 `a_n * x^n + a_{n-1} * x^{n-1} + ... + a_0` 和 `b_m * x^m + b_{m-1} * x^{m-1} + ... + b_0` 相加,其中 `n` 和 `m` 分别表示第一个多项式的最高次幂和第二个多项式的最高次幂)相加:
```c
#include <stdio.h>
// 定义一元多项式结构体
typedef struct {
int coefficient; // 系数
int exponent; // 指数
} Polynomial;
// 将两个多项式相加
void addPolynomials(Polynomial* poly1, Polynomial* poly2, Polynomial* result) {
int maxDegree = (poly1->exponent > poly2->exponent) ? poly1->exponent : poly2->exponent;
for(int i = 0; i <= maxDegree; ++i) {
if(i == poly1->exponent)
result->coefficient += poly1->coefficient;
else if(i == poly2->exponent)
result->coefficient += poly2->coefficient;
else
result->coefficient += 0; // 如果不是最高次项,则系数为0
result->exponent = i; // 更新结果多项式的指数
}
}
// 示例用法
int main() {
Polynomial p1 = {5, 2}; // 5x^2
Polynomial p2 = {3, 1}; // 3x^1
Polynomial sum;
addPolynomials(&p1, &p2, &sum); // 相加
printf("Sum of %dx^%d and %dx^%d is %dx^%d\n",
p1.coefficient, p1.exponent, p2.coefficient, p2.exponent,
sum.coefficient, sum.exponent);
return 0;
}
```
在这个示例中,我们首先计算两个多项式的最大次数,然后遍历从0到这个最大次数的指数,对每个指数对应的系数进行累加。最后,打印出相加后的多项式。
阅读全文
相关推荐
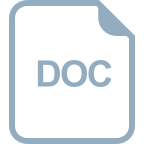
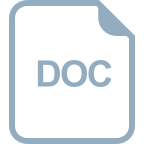
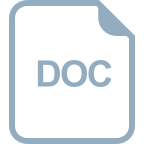
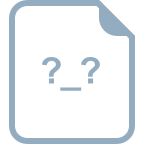







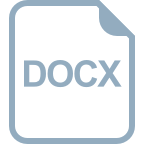





