定义一个主函数main来测试单链表里面头插法createlistf,尾插法createlistr,查找locateelem,插入listinsert,删除listdelete
时间: 2024-10-03 12:02:57 浏览: 26
在大多数编程语言中,特别是像C++这样的命令行应用程序中,你可以创建一个名为`main`的函数作为程序的入口点来测试链表的各种操作。这里是一个简化的示例,展示了如何使用`main`函数来测试你提到的链表操作:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点结构体和相应的函数
struct ListNode {
int data;
ListNode* next;
};
ListNode* createlistf(int first); // 头插法创建链表
ListNode* createlistr(int last); // 尾插法创建链表
bool locateelem(ListNode* head, int value); // 查找元素
void listinsert(ListNode*& head, int value); // 在链表中插入元素
ListNode* listdelete(ListNode*& head, int value); // 删除链表中的元素
int main() {
// 初始化链表
ListNode* head = nullptr;
// 测试头插法
cout << "Testing createListF with value 1:\n";
head = createlistf(1);
if (head) {
cout << "Head of the created list: " << head->data << endl;
} else {
cout << "Failed to create the list." << endl;
}
// 测试尾插法
cout << "\nTesting createListR with value 2:\n";
ListNode* tail = createlistr(2);
if (tail && tail->next == nullptr) {
cout << "Tail of the created list: " << tail->data << endl;
} else {
cout << "Failed to create the list or append element." << endl;
}
// 测试其他功能
cout << "\nInserting value 3 at the beginning:\n";
listinsert(head, 3);
if (locateelem(head, 3)) {
cout << "Element 3 is present in the list.\n";
} else {
cout << "Element 3 not found after insertion.\n";
}
// 然后尝试删除元素
cout << "\nDeleting value 2 from the list:\n";
head = listdelete(head, 2);
if (locateelem(head, 2)) {
cout << "Value 2 should have been deleted but found.\n";
} else {
cout << "Value 2 successfully deleted.\n";
}
return 0; // 结束main函数
}
阅读全文
相关推荐
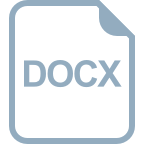
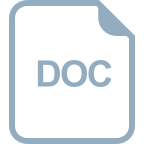
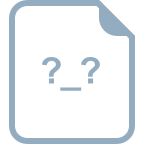















