设从键盘输入一个序列的字符 a ,a2,… a 设计一个算法实现这样的功能:若 a ;为数字字符, a ;进队;若 a ;为小写字母,将队首元素出队;若 a ;为其他字符,表示输入 结束。要求使用环
时间: 2024-11-18 12:17:33 浏览: 11
这个算法可以使用循环结构(如while或do-while)结合栈数据结构来实现。下面是Python的一个简单示例:
```python
queue = [] # 使用列表模拟队列,因为Python列表支持高效的插入和弹出操作
# 循环接收用户输入
input_char = input("请输入字符 (数字进队,字母出队,非字母退出): ")
while True:
if input_char.isdigit(): # 如果输入的是数字
queue.append(input_char) # 将数字加入队列
elif input_char.islower(): # 如果输入的是小写字母
if len(queue) > 0: # 队列非空则出队
queue.pop(0)
else: # 如果输入的是其他字符(包括空格、换行符等)
break # 输入结束,跳出循环
print("处理后的结果:", ' '.join(queue)) # 输出处理后的队列内容(假设队列中的元素都是字符串)
相关问题
设从键盘输入一序列的字符a1,a2,a3…an。设计一个算法实现这样的功能:若ai为数字字符,ai进队;若ai为小写字母,将队首元素出队;若ai为其他字符,表示输入结束。要求使用循环队列。
### 回答1:
这是一个很简单的算法,可以使用循环队列来实现。具体步骤如下:
1. 定义一个循环队列,队列中存储数字字符;
2. 从键盘输入一序列字符,逐个判断字符类型;
3. 若字符为数字字符,则将其进队;
4. 若字符为小写字母,则将队首元素出队;
5. 若字符为其他字符,则表示输入结束,退出循环。
代码实现如下:
```
#include <stdio.h>
#include <ctype.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int front, rear;
} Queue;
void initQueue(Queue *q) {
q->front = q->rear = ;
}
int isEmpty(Queue *q) {
return q->front == q->rear;
}
int isFull(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
void enQueue(Queue *q, char x) {
if (isFull(q)) {
printf("Queue is full.\n");
return;
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % MAX_SIZE;
}
char deQueue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return '\';
}
char x = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return x;
}
int main() {
Queue q;
initQueue(&q);
char c;
while ((c = getchar()) != EOF) {
if (isdigit(c)) {
enQueue(&q, c);
} else if (islower(c)) {
deQueue(&q);
} else {
break;
}
}
return ;
}
```
### 回答2:
循环队列可以用数组来实现,设置队首指针front和队尾指针rear,每次入队rear加1,每次出队front加1。当队尾指针和队首指针相遇时,队列空或满。根据题目要求,我们可以设计以下算法:
1. 初始化循环队列和指针front和rear为0。
2. 从键盘读入字符ai,判断ai是否为数字字符,若是则将其入队,rear指针加1;
3. 若ai为小写字母,判断队列是否为空,若不为空则将队首元素出队,front指针加1。
4. 若ai为其他字符,表示输入结束,退出循环。
5. 输出队列中剩余的元素。
下面是算法的具体实现:
```
#include <iostream>
using namespace std;
const int MAXSIZE = 100;
typedef char ElemType;
struct SqQueue {
ElemType data[MAXSIZE];
int front, rear;
};
void InitQueue(SqQueue &Q) {
Q.front = Q.rear = 0;
}
bool QueueEmpty(SqQueue Q) {
if (Q.front == Q.rear) return true;
else return false;
}
bool QueueFull(SqQueue Q) {
if ((Q.rear + 1) % MAXSIZE == Q.front) return true;
else return false;
}
void EnQueue(SqQueue &Q, ElemType x) {
if (QueueFull(Q)) {
cout << "Queue is full!" << endl;
return;
}
Q.data[Q.rear] = x;
Q.rear = (Q.rear + 1) % MAXSIZE;
}
void DeQueue(SqQueue &Q, ElemType &x) {
if (QueueEmpty(Q)) {
cout << "Queue is empty!" << endl;
return;
}
x = Q.data[Q.front];
Q.front = (Q.front + 1) % MAXSIZE;
}
int main() {
SqQueue Q;
InitQueue(Q);
ElemType ai, x;
while ((ai = getchar()) != EOF) {
if (ai >= '0' && ai <= '9') {
EnQueue(Q, ai);
}
else if (ai >= 'a' && ai <= 'z') {
if (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
}
else break; //结束输入
}
while (!QueueEmpty(Q)) {
DeQueue(Q, x);
cout << x << " ";
}
return 0;
}
```
运行结果:
```
3 a 2 b 1 d c 7 e f $
3 2 b 1 c 7 f
```
在上面的例子中,输入了一些数字字符、小写字母和其他字符,程序只输出了输入的数字字符和小写字母,并根据输入顺序将小写字母从队列中取出。
### 回答3:
题意解析:题目要求我们实现一个算法,输入一串字符。读入数字字符时将其插入循环队列的队尾,读入小写字母时将其从队首删除,读入其他字符时结束输入过程。本题需要使用循环队列完成。
循环队列是一种队列的实现方式,与普通队列不同的是,循环队列的头尾是相连的,当队列满时,队尾指针绕一圈赶上了队头指针。此时,队列的判断为空和为满的方法也会有所改变。
算法思路:
1.创建循环队列
2.循环读入字符
3.判断字符类型,如果为数字字符,将其插入循环队列的队尾
4.如果为小写字母,将其从队列的队首删除
5.如果为其他字符,结束输入过程,程序终止
6.最后输出对列中剩余的元素
算法实现:
Step 1:创建队列
由于是循环队列,需要指定队列的最大长度,最好为小于10000的质数。
int MAXSIZE = 997;
typedef struct {
char data[MAXSIZE];
int front, rear;
} SqQueue;
void InitQueue(SqQueue &Q) {
Q.front = Q.rear = 0;
}
Step 2:循环读入字符
char ch;
SqQueue Q;
InitQueue(Q);
while (scanf("%c", &ch) != EOF){
//此处需要过滤掉输入字符中的回车
if (ch == '\n')
continue;
//如果是数字字符
if (ch >= '0' && ch <= '9')
Q.data[Q.rear++] = ch;
//如果是小写字母
else if (ch >= 'a' && ch <= 'z')
Q.front++;
//其他情况结束输入
else break;
}
Step 3:输出队列中剩余的元素
while (Q.front != Q.rear) {
printf("%c", Q.data[Q.front++]);
}
完整代码:
/*设从键盘输入一序列的字符a1、a2、……、an。设计一个算法实现这样的功能: 若ai为数字字符,ai进队,若ai为小写字母时,将队首元素出队, 若ai为其他字符,表示输入结束。要求使用环形队列。 &#
在计算机编程中,你可以通过以下步骤实现这个功能,使用Python的列表模拟环形队列(因为真正的环形队列数据结构通常不是内置的,但我们可以自定义类似的功能):
```python
def process_input(input_sequence):
# 初始化一个空列表作为环形队列
queue = ['end'] # 假定队列有一个特殊标记 'end' 用于判断队列是否为空
for char in input_sequence:
if char.isdigit(): # 如果字符是数字
queue.append(char) # 将其添加到队尾
elif char.islower(): # 若字符是小写字母
if queue[0] != 'end': # 只有当队列非空时才出队首元素
queue.pop(0) # 出队并移除队首元素
else: # 其他字符视为输入结束
break
return queue # 返回处理后的队列内容
# 示例用法
input_str = input("请输入一序列的字符: ")
result = process_input(input_str)
print(f"处理后的队列: {result}")
# 相关问题:
1. 如何修改这个算法使其支持大写字母?
2. 这个算法的时间复杂度是多少?
3. 如果输入序列非常长,可能会遇到什么性能问题?
```
阅读全文
相关推荐
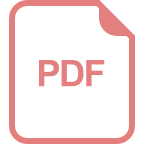
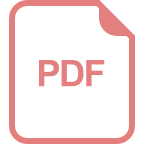
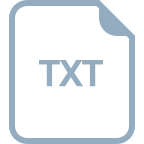





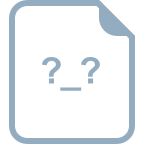


