pthread_create创建线程并绑定到硬核6的示例代码
时间: 2024-10-23 18:12:32 浏览: 43
在C语言中,`pthread_create`函数用于创建新的线程,并可以指定线程的优先级、堆栈大小等参数。如果想要创建一个线程并且将其绑定到特定的CPU核心(比如第6核),这在现代多核处理器上通常不可直接通过`pthread_create`实现,因为操作系统默认的线程调度策略通常是动态的,难以直接绑定。
但是,你可以使用Linux提供的`sched_setaffinity`系统调用来设置线程的亲缘性集合(Sibling Affinity),该集合指定了线程愿意运行在其兄弟(在同一CPU组内的其他线程)旁边。以下是一个简单的例子:
```c
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
#include <sched.h>
void *thread_function(void *arg) {
// 线程主函数
printf("Thread running on CPU %d\n", sched_getcpu());
// 这里假设已经设置了线程的亲缘性集合
return NULL;
}
int main() {
pthread_t thread_id;
cpu_set_t cpuset;
// 初始化cpuset,这里仅包含CPU 6
CPU_ZERO(&cpuset);
CPU_SET(5, &cpuset); // 注意,索引从0开始,所以5对应CPU 6
if (pthread_create(&thread_id, NULL, thread_function, NULL)) {
perror("Failed to create thread");
return -1;
}
// 尝试将线程绑定到cpuset
if (pthread_setaffinity_np(thread_id, sizeof(cpuset), &cpuset) != 0) {
perror("Failed to set affinity");
pthread_cancel(thread_id); // 如果失败,取消线程
return -1;
}
// 等待线程结束
pthread_join(thread_id, NULL);
return 0;
}
```
注意:实际操作中,能否成功将线程绑定到特定核心取决于系统的线程调度策略以及是否有足够的上下文切换开销。另外,在某些平台上,这种硬编码的CPU绑定可能会受限或者不可行。
阅读全文
相关推荐
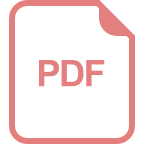
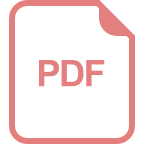
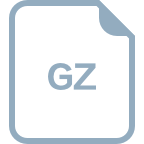















