使用pthread_create()创建线程
时间: 2023-07-25 13:26:40 浏览: 42
使用pthread_create()函数可以创建一个新的线程,函数原型如下:
```
int pthread_create(pthread_t* thread, const pthread_attr_t* attr, void* (*start_routine) (void*), void* arg);
```
其中,参数thread是指向线程标识符的指针,attr是指向线程属性的指针,start_routine是指向线程函数的指针,arg是传递给线程函数的参数。
调用pthread_create()函数后,会创建一个新的线程,并在新线程中执行start_routine函数。线程的标识符会被存储在thread指向的内存中。如果创建成功,函数返回0;否则,返回一个错误码。需要注意的是,创建线程前必须先初始化线程属性,否则可以将attr参数设置为NULL。
以下是一个简单的示例代码,演示了如何使用pthread_create()创建一个新的线程:
```
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
void* thread_func(void* arg) {
printf("Hello from thread!\n");
pthread_exit(NULL);
}
int main() {
pthread_t tid;
int ret = pthread_create(&tid, NULL, thread_func, NULL);
if (ret != 0) {
printf("Failed to create thread!\n");
exit(EXIT_FAILURE);
}
printf("Hello from main!\n");
pthread_join(tid, NULL);
return 0;
}
```
在该示例代码中,主函数中调用pthread_create()函数创建一个新的线程,并将线程函数指针设置为thread_func。线程函数thread_func会输出一条信息,然后调用pthread_exit()函数退出线程。主函数中也输出一条信息,并且调用pthread_join()函数等待新线程结束。
相关推荐
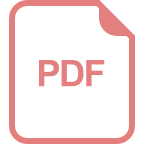
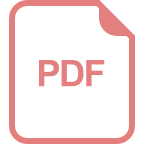
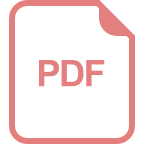














