c++俄罗斯方块 完整源代码
时间: 2023-12-07 11:04:55 浏览: 128
以下是C++实现俄罗斯方块的完整源代码,包括方块的生成、移动、旋转、消除等功能:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <conio.h>
#include <windows.h>
using namespace std;
const int WIDTH = 10; // 游戏区域宽度
const int HEIGHT = 20; // 游戏区域高度
const int BLOCK_SIZE = 4; // 方块大小
const int SHAPE_TYPES = 7; // 方块类型数目
// 方块类型
const int SHAPES[SHAPE_TYPES][BLOCK_SIZE][BLOCK_SIZE] = {
// I型
{
{0, 0, 0, 0},
{1, 1, 1, 1},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// J型
{
{1, 0, 0, 0},
{1, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// L型
{
{0, 0, 1, 0},
{1, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// O型
{
{1, 1, 0, 0},
{1, 1, 0, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// S型
{
{0, 1, 1, 0},
{1, 1, 0, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// T型
{
{0, 1, 0, 0},
{1, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
},
// Z型
{
{1, 1, 0, 0},
{0, 1, 1, 0},
{0, 0, 0, 0},
{0, 0, 0, 0}
}
};
// 方块颜色
const int COLORS[SHAPE_TYPES] = {
FOREGROUND_BLUE | FOREGROUND_GREEN | FOREGROUND_INTENSITY, // I型
FOREGROUND_BLUE | FOREGROUND_INTENSITY, // J型
FOREGROUND_RED | FOREGROUND_INTENSITY, // L型
FOREGROUND_GREEN | FOREGROUND_INTENSITY, // O型
FOREGROUND_BLUE | FOREGROUND_GREEN | FOREGROUND_INTENSITY, // S型
FOREGROUND_RED | FOREGROUND_GREEN | FOREGROUND_INTENSITY, // T型
FOREGROUND_RED | FOREGROUND_GREEN | FOREGROUND_INTENSITY // Z型
};
// 方块结构体
struct Block {
int x, y; // 方块左上角坐标
int shape; // 方块类型
int angle; // 方块旋转角度
int color; // 方块颜色
};
// 游戏区域
int board[HEIGHT][WIDTH] = {0};
// 生成新方块
Block newBlock() {
Block block;
block.x = WIDTH / 2 - 2;
block.y = 0;
block.shape = rand() % SHAPE_TYPES;
block.angle = 0;
block.color = COLORS[block.shape];
return block;
}
// 绘制方块
void drawBlock(Block block, bool clear = false) {
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
if (SHAPES[block.shape][i][j] != 0) {
int x = block.x + j;
int y = block.y + i;
if (x >= 0 && x < WIDTH && y >= 0 && y < HEIGHT) {
COORD pos = {x * 2, y};
SetConsoleCursorPosition(hConsole, pos);
if (clear) {
cout << " ";
} else {
SetConsoleTextAttribute(hConsole, block.color);
cout << "■";
}
}
}
}
}
}
// 检查方块是否与游戏区域重叠
bool checkOverlap(Block block) {
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
if (SHAPES[block.shape][i][j] != 0) {
int x = block.x + j;
int y = block.y + i;
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT || board[y][x] != 0) {
return true;
}
}
}
}
return false;
}
// 将方块放入游戏区域
void placeBlock(Block block) {
for (int i = 0; i < BLOCK_SIZE; i++) {
for (int j = 0; j < BLOCK_SIZE; j++) {
if (SHAPES[block.shape][i][j] != 0) {
int x = block.x + j;
int y = block.y + i;
if (x >= 0 && x < WIDTH && y >= 0 && y < HEIGHT) {
board[y][x] = block.color;
}
}
}
}
}
// 消除满行
void clearLines() {
int lines = 0;
for (int i = HEIGHT - 1; i >= 0; i--) {
bool full = true;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
full = false;
break;
}
}
if (full) {
lines++;
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
board[k][j] = board[k - 1][j];
}
}
for (int
阅读全文
相关推荐
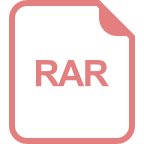
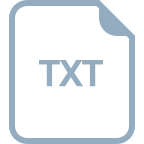
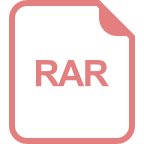
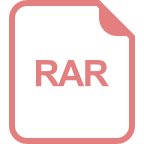
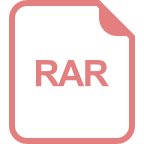
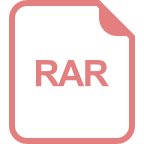
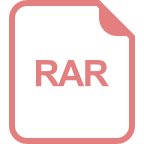
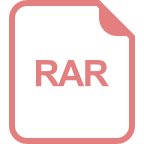
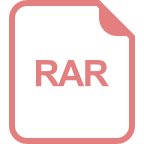
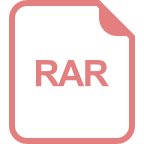

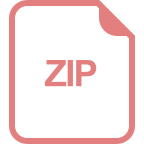